Spring Security Java Config - custom AuthenticationProvider and UserDetailsService
Here is an example of customized AuthenticationProvider and customized UserDetailsService:
@Configuration
@EnableWebMvcSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
public void registerGlobalAuthentication(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(customAuthenticationProvider());
}
@Bean
AuthenticationProvider customAuthenticationProvider() {
CustomAuthenticationProvider impl = new CustomAuthenticationProvider();
impl.setUserDetailsService(customUserDetailsService());
/* other properties etc */
return impl ;
}
@Bean
UserDetailsService customUserDetailsService() {
/* custom UserDetailsService code here */
}
}
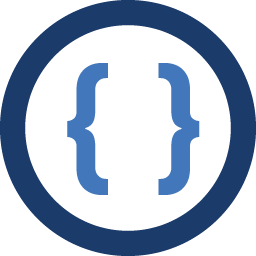
Admin
Updated on July 03, 2022Comments
-
Admin almost 2 years
I use java configuration to configure Spring Security, and I have customized AuthenticationProvider and customized UserDetailsService, to add extra login field following http://forum.spring.io/forum/spring-projects/security/95715-extra-login-fields
I have difficulty to add both of the customized Classes into Spring Security framework by using java configuration. As java doc of AuthenticationProvider#authenticationProvider describes:
Add authentication based upon the custom AuthenticationProvider that is passed in. Since the AuthenticationProvider implementation is unknown, all customizations must be done externally and the AuthenticationManagerBuilder is returned immediately.
This method does NOT ensure that the UserDetailsService is available for the getDefaultUserDetailsService() method.
So my question is what is the approach to set UserDetailsService in this case?
-
Admin almost 9 yearsI notice that you initialize your
customAuthenticationProvider
andcustomUserDetailsService
manually, is it not better to@Autowired
them directly? -
Ritesh almost 9 years
@Autowired
is used in@Configuration
class when you are wiring outside beans. In this code,customAuthenticationProvider
andcustomUserDetailsService
beans are declared in the same class and so there is no use case for@Autowired
. Also note thatAuthenticationManagerBuilder
is declared somewhere else and so it is ok to use@Autowired
. -
user3791111 almost 7 yearsimpl.setUserDetailsService(customUserDetailsService()); // this will not work, - the AuthenticationProvider interface has no setUserDetailsService() method.
-
Ritesh almost 7 yearsSince it is a custom AuthenticationProvider implementation, you can add this method (after all any authentication provider would need a mechanism to load user details). See implementations of
AuthenticationProvider
such as DaoAuthenticationProvider, which has thesetUserDetailsService
method.