Springfox swagger not working in spring boot 2.2.0
Solution 1
Fixed!!!
The issue exists as spring fox libraries internally depend on spring-plugin-core:1.2.0 but it actually requires spring-plugin-core:2.0.0. This dependency correction seems to be missing in the SNAPSHOT version of the swagger libraries for supporting web flux.
We just need to correct Maven dependencies as given below and no code change is required:
- Maven POM Dependencies fix
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>3.0.0-SNAPSHOT</version>
<exclusions>
<exclusion>
<groupId>org.springframework.plugin</groupId>
<artifactId>spring-plugin-core</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>3.0.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-spring-webflux</artifactId>
<version>3.0.0-SNAPSHOT</version>
<exclusions>
<exclusion>
<groupId>org.springframework.plugin</groupId>
<artifactId>spring-plugin-core</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.plugin</groupId>
<artifactId>spring-plugin-core</artifactId>
<version>2.0.0.RELEASE</version>
</dependency>
- Swagger Configuration: (Same as before just for reference)
@Configuration
@EnableSwagger2WebFlux
public class SwaggerConfiguration {
@Bean
public Docket api(final TypeResolver typeResolver) {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
Solution 2
You can use SpringFox 3.0.0-SNAPSHOT
with Spring Boot 2.2.X
- Since it is a SNAPSHOT version, it is not published in the official repository of maven and needs to be pulled from jcenter-snapshots
-
@EnableSwagger2
is removed and changed to@EnableSwagger2WebMvc
and@EnableSwagger2WebFlux
Maven
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>3.0.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>3.0.0-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-data-rest</artifactId>
<version>3.0.0-SNAPSHOT</version>
</dependency>
Repository
<repositories>
<repository>
<id>jcenter-snapshots</id>
<name>jcenter</name>
<url>http://oss.jfrog.org/artifactory/oss-snapshot-local/</url>
</repository>
</repositories>
Sample Configuration
@EnableSwagger2WebMvc
@Import(SpringDataRestConfiguration.class)
public class SpringFoxConfig {
//...
}
References
Note: It seems SpringFox
is not dead.
Solution 3
Apparently, since SpringFox
is abandoned and its last version is no long compatible with Spring Boot 2.2.X, you will have to look for alternatives (see issue reported in Github here).
One possible option is to use OpenAPI docs
. For that you need to remove the SpringFox
dependencies and add these:
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>1.2.15</version>
</dependency>
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-webmvc-core</artifactId>
<version>1.2.15</version>
</dependency>
Also, you will have to remove the Swagger
configuration class and provide this:
@Configuration
public class OpenApiConfig {
@Bean
public OpenAPI customOpenAPI() {
return new OpenAPI()
.components(new Components())
.info(new Info().title("Contact Application API").description(
"This is a sample Spring Boot RESTful service using springdoc-openapi and OpenAPI 3."));
}
}
In my case, everything seems to work with the exception of the JPA Rest repositories that are not being exposed. I will update this answer with a fix, if I find one.
These are some of the links I was checking to fix this:
Solution 4
I upgraded to Springfox Swagger2 to 3.0.0 version and spring plugin code to 2.0.0.RELEASE version. It worked for me.
- Spring Boot Version - 2.3.9.RELEASE
<dependency>
<groupId>org.springframework.plugin</groupId>
<artifactId>spring-plugin-core</artifactId>
<version>2.0.0.RELEASE</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>3.0.0</version>
</dependency>
Solution 5
Remove HATEOS dependency, but add only swagger. It works
// Using only the swagger as of now, since hateos is not updated the fix for Spring 2.1.X and above
compile group: 'io.springfox', name: 'springfox-swagger-ui', version: '2.9.2'
compile group: 'io.springfox', name: 'springfox-swagger2', version: '2.9.2'
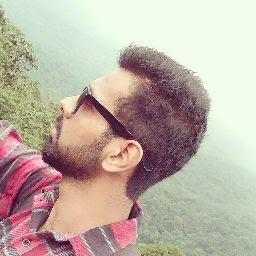
Joyson Rego
Updated on December 28, 2021Comments
-
Joyson Rego over 2 years
I want to upgrade spring boot v2.1.9 to 2.2.0. But after the upgrade I am getting some exceptions, which says spring fox is using an older version of spring-plugin-core.
Is there any alternate solution for this or do I need to give up the springfox plugin?
*************************** APPLICATION FAILED TO START *************************** Description: An attempt was made to call a method that does not exist. The attempt was made from the following location: springfox.documentation.spring.web.plugins.DocumentationPluginsManager.createContextBuilder(DocumentationPluginsManager.java:152) The following method did not exist: org.springframework.plugin.core.PluginRegistry.getPluginFor(Ljava/lang/Object;Lorg/springframework/plugin/core/Plugin;)Lorg/springframework/plugin/core/Plugin; The method's class, org.springframework.plugin.core.PluginRegistry, is available from the following locations: jar:file:/C:/Users/regosa/.m2/repository/org/springframework/plugin/spring-plugin-core/2.0.0.RELEASE/spring-plugin-core-2.0.0.RELEASE.jar!/org/springframework/plugin/core/PluginRegistry.class It was loaded from the following location: file:/C:/Users/regosa/.m2/repository/org/springframework/plugin/spring-plugin-core/2.0.0.RELEASE/spring-plugin-core-2.0.0.RELEASE.jar Action: Correct the classpath of your application so that it contains a single, compatible version of org.springframework.plugin.core.PluginRegistry
maven dependency tree file : https://drive.google.com/file/d/1gayvvVe_VsB1P2Hi2rcwRw8NdK89qtbq/view
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.xxxxx.business.workflow</groupId> <artifactId>xxxxx-component-workflow-starter</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>xxxxx-component-workflow-starter</name> <description>xxxxx-component-workflow-starter</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.0.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>11</java.version> <jaxb-runtime.version>2.4.0-b180830.0438</jaxb-runtime.version> <spring-cloud.version>Greenwich.M1</spring-cloud.version> <zeebe-version>0.20.1</zeebe-version> <google-guava.version>27.0.1-jre</google-guava.version> <xxxxx.version>1.0</xxxxx.version> <swagger.version>2.9.2</swagger.version> <jjwt.version>0.9.1</jjwt.version> <json.version>20180813</json.version> <slf4j-api.version>1.7.25</slf4j-api.version> <mysql.version>8.0.11</mysql.version> <mongo-java-driver.version>3.10.1</mongo-java-driver.version> <commons-io.version>2.6</commons-io.version> <commons-lang.version>2.6</commons-lang.version> <commons-pool2.version>2.5.0</commons-pool2.version> <redis.version>3.1.0</redis.version> <velocity.version>1.7</velocity.version> <velocity-tools.version>2.0</velocity-tools.version> <logstash-logback-encoder.version>5.3</logstash-logback-encoder.version> <httpclient.version>4.5.6</httpclient.version> <jaxb-runtime.version>2.4.0-b180830.0438</jaxb-runtime.version> <env>local</env> </properties> <dependencies> <!-- Start: Spring Libraries --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-rest</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> <dependency> <groupId>org.springframework.kafka</groupId> <artifactId>spring-kafka</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-sleuth</artifactId> </dependency> <!-- End: Spring Libraries --> <!-- Adding JAXB Runtime since it is not shipped with JDK 9+ --> <dependency> <groupId>org.glassfish.jaxb</groupId> <artifactId>jaxb-runtime</artifactId> <version>${jaxb-runtime.version}</version> </dependency> <!-- Start: xxxxx Libraries --> <dependency> <groupId>com.xxxxx</groupId> <artifactId>xxxxx-entity</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx</groupId> <artifactId>xxxxx-redis</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx</groupId> <artifactId>xxxxx-mongo</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx</groupId> <artifactId>xxxxx-util</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx.model</groupId> <artifactId>xxxxx-model</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx.service</groupId> <artifactId>xxxxx-common-service</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx.service</groupId> <artifactId>xxxxx-common-messaging</artifactId> <version>${xxxxx.version}</version> </dependency> <dependency> <groupId>com.xxxxx.service</groupId> <artifactId>xxxxx-common-security</artifactId> <version>${xxxxx.version}</version> </dependency> <!-- End: xxxxx Libraries --> <!-- Adding Zeebe client as part of the Spring Startup --> <dependency> <groupId>io.zeebe</groupId> <artifactId>zeebe-client-java</artifactId> <version>${zeebe-version}</version> </dependency> <!-- https://mvnrepository.com/artifact/com.google.guava/guava --> <dependency> <groupId>com.google.guava</groupId> <artifactId>guava</artifactId> <version>${google-guava.version}</version> </dependency> <!-- Logstash Log Encoder --> <dependency> <groupId>net.logstash.logback</groupId> <artifactId>logstash-logback-encoder</artifactId> <version>${logstash-logback-encoder.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.mongodb/mongo-java-driver --> <dependency> <groupId>org.mongodb</groupId> <artifactId>mongo-java-driver</artifactId> <version>${mongo-java-driver.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/commons-io/commons-io --> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>${commons-io.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/commons-lang/commons-lang --> <dependency> <groupId>commons-lang</groupId> <artifactId>commons-lang</artifactId> <version>${commons-lang.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.commons/commons-pool2 --> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-pool2</artifactId> <version>${commons-pool2.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/redis.clients/jedis --> <dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>${redis.version}</version> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>${httpclient.version}</version> </dependency> <!-- Start: Swagger Libraries --> <!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger2 --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>${swagger.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/io.springfox/springfox-swagger-ui --> <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>${swagger.version}</version> </dependency> <!-- End: Swagger Libraries --> <!-- Start: Spring Boot and Security Test Libraries --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!-- End: Spring Boot and Security Test Libraries --> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <finalName>xxxxx-component-workflow-starter</finalName> <filters> <filter>${env}-build.properties</filter> </filters> <resources> <resource> <filtering>true</filtering> <directory>${project.basedir}/src/main/resources</directory> </resource> </resources> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.7.0</version> <configuration> <release>${java.version}</release> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <configuration> <archive> <manifestEntries> <Automatic-Module-Name>com.xxxxx.business.workflow.component.starter</Automatic-Module-Name> </manifestEntries> </archive> </configuration> </plugin> </plugins> </build> <repositories> <repository> <id>spring-milestones</id> <name>Spring Milestones</name> <url>https://repo.spring.io/milestone</url> <snapshots> <enabled>false</enabled> </snapshots> </repository> </repositories> </project>