SQLAlchemy NameError: Name 'db' is not defined (?)
Solution 1
you can make a db.py where you can store the code db = SQLAlchemy()
. Then import in in app.py. now you can able to call db. or just remove APP
in db=SQLAlchemy(app)
Solution 2
I think you probably need to run some of the other code first so that you define db
and your table schema. Then you can run db.create_all()
.
from flask import Flask, render_template, request
from flask.ext.sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config(['SQLALCHEMY_DATABASE_URI'] =
'postgresql://postgres:[email protected]/height_collector')
db = SQLAlchemy(app)
class Data(db.Model):
__tablename__ = 'data'
id = db.Column(db.Integer, primary_key=True)
email_ = db.Column(db.String(120), unique=True)
height_ = db.Column(db.Integer)
def __init__(self, email_, height_):
self.email_ = email_
self.height_ = height_
db.create_all()
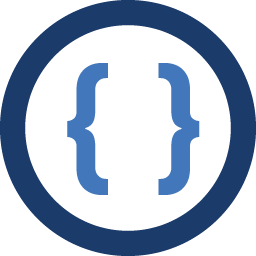
Admin
Updated on June 05, 2022Comments
-
Admin 12 months
I am working on a Udemy course using flask to record heights. I am at the point where we are using PostgreSQL, and I have it installed, and I have his code copied exactly:
from flask import Flask, render_template, request from flask.ext.sqlalchemy import SQLAlchemy app=Flask(__name__) app.config(['SQLALCHEMY_DATABASE_URI']='postgresql://postgres:password @localhost/height_collector') db=SQLAlchemy(app) class Data(db.Model): __tablename__='data' id=db.Column(db.Integer, primary_key=True) email_=db.Column(db.String(120), unique=True) height_=db.Column(db.Integer) def __init__(self, email_, height_): self.email_=email_ self.height_=height_ @app.route("/") def index(): return render_template("index.html") @app.route("/success", methods=["post"]) def success(): if request.method=='POST': email=request.form['email_name'] height=request.form['height_name'] print(height,email) return render_template("success.html") if __name__=='__main__': app.debug=True app.run()
Problem comes into play, when he says to run python in a virtual env, and then enter :db.create_all() to create a database in PostgreSQL and I get this error : File <'stdin'>, line 1 in NameError: Name 'db' is not defined
Not sure how to proceed, any input would be appreciated.
-
Zani Kastrati about 2 yearsIs everything OK? This way it worked for me!