SQLALCHEMY_DATABASE_URI not set
Solution 1
You probably need to put this line app.config['SQLALCHEMY_DATABASE_URI'] = "mysql..."
before the SQLAlchemy(app)
instanciation.
Another option is to create SQLAlchemy()
without parametters, to configure URI, and finally to tell SQLAlchemy
to link with your app via sqldb.init_app(app)
Note that it is what you've done in your create_app
function, but you never use it ?
Solution 2
Like the answer above, if you use it this way, change
sqldb = SQLAlchemy(app)
to
sqldb = SQLAlchemy()
Solution 3
I got the same error, and it was gone after setting the FLASK_APP environment variable :
export FLASK_APP=run.py
Start the application :
flask run --host=0.0.0.0 --port=5000
Solution 4
This type of error occurs when app.config['SQLALCHEMY_TRACK_MODIFICATIONS']
is not set. You can set it as True
or False
like so:
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
or
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = True
Related videos on Youtube
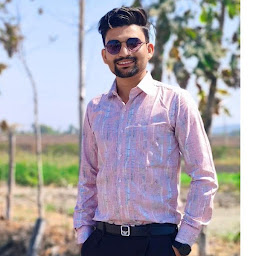
Kaushik Makwana
Updated on November 21, 2021Comments
-
Kaushik Makwana over 2 years
I tried to work with
CURD
operation usingFlask
andSQLAlchemy
.But gettingError
while connecting to database.Here is the
Error
log./usr/local/lib/python3.4/dist-packages/flask_sqlalchemy/__init__.py:819: UserWarning: SQLALCHEMY_DATABASE_URI not set. Defaulting to "sqlite:///:memory:". 'SQLALCHEMY_DATABASE_URI not set. Defaulting to ' /usr/local/lib/python3.4/dist-packages/flask_sqlalchemy/__init__.py:839: FSADeprecationWarning: SQLALCHEMY_TRACK_MODIFICATIONS adds significant overhead and will be disabled by default in the future. Set it to True or False to suppress this warning. 'SQLALCHEMY_TRACK_MODIFICATIONS adds significant overhead and '
here is my code & setup
# database.py from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) sqldb = SQLAlchemy(app) app.config['SQLALCHEMY_DATABASE_URI'] = "mysql+pymysql://root:root@localhost/myDbName" # create app def create_app(): sqlDB = SQLAlchemy(app) sqlDB.init_app(app) sqlDB.create_all() return app
here is models.py
from ..database import create_app sqldb = create_app() # Users Model class Users(sqldb.Model): __tablename__ = 'users' id = sqldb.Column(sqldb.Integer, primary_key = True) db = sqldb.Column(sqldb.String(40)) def __init__(self,email,db): self.email = email self.db = db def __repr__(self,db): return '<USER %r>' % self.db
here is routes.py
# Import __init__ file from __init__ import app import sys # JSON from bson import dumps # login @app.route('/', methods = ['GET']) def login(): try: # import users model from Model.models import Users,sqldb sqldb.init_app(app) sqldb.create_all() getUser = Users.query.all() print(getUser) return 'dsf' except Exception as e: print(e) return "error."
-
Kaushik Makwana about 7 yearsThanks @B. Barbieri :) now error is gone but there is come new error. The sqlalchemy extension was not registered to the current application. Please make sure to call init_app() first.
-
B. Barbieri about 7 yearsIt's not really clear where this comes from, mainly because your imports are quite a mess ... You probably need to remove this
create_app
function, and to make all your imports fromdatabase.py
: this will prevent you from trying to usesqldb
before it's fully configured.