Static files won't load when out of debug in Django
Solution 1
Static files app is not serving static media automatically in DEBUG=False
mode. From django.contrib.staticfiles.urls
:
# Only append if urlpatterns are empty
if settings.DEBUG and not urlpatterns:
urlpatterns += staticfiles_urlpatterns()
You can serve it by appending to your urlpatterns
manually or use a server to serve static files (like it is supposed to when running Django projects in non-DEBUG mode).
Though one thing I am wondering is why you get a 500 status code response instead of 404. What is the exception in this case?
EDIT
So if you still want to serve static files via the staticfiles app add the following to your root url conf (urls.py
):
if settings.DEBUG is False: #if DEBUG is True it will be served automatically
urlpatterns += patterns('',
url(r'^static/(?P<path>.*)$', 'django.views.static.serve', {'document_root': settings.STATIC_ROOT}),
)
Some things you need to keep in mind though:
- don't use this on a production environment (its slower since static files rendering goes through Django instead served by your web server directly)
- most likely you have to use management commands to collect static files into your
STATIC_ROOT
(manage.py collectstatic
). See the staticfiles app docs for more information. This is simply necessary since you run on non-Debug mode. - don't forget
from django.conf import settings
in yoururls.py
:)
Solution 2
In Django 1.3, if you are just testing using the manage.py runserver, you can add the option "--insecure", as described in the staticfiles docs:
It seems to still send emails to the admin saying that there is no template, but it does serve static files.
I'm not sure if the email issue is on purpose or a bug
Related videos on Youtube
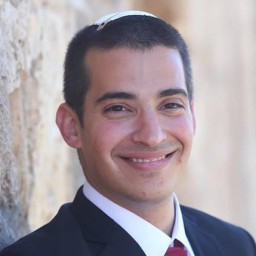
Nachshon Schwartz
Fullstack Developer at Wix. Previously a web developer in the IDF, developing a Play Framework, Angular business process monitoring web application. Technion, Software Engineering** graduate from Israel. Programming languages: Javascript, Java, Ruby,C++ Python. DB: Neo4J, OracleDB, MongoDB Web Frameworks: Play Framework, Angular, Ruby on Rails, Django Some of my work: Cherrity - Cherrity is a website I created during my 4th year - Yearly Project with a team of 7 other Software Engineering students. It's a Dynamic website for organizing charity projects.@nayish
Updated on June 04, 2022Comments
-
Nachshon Schwartz over 1 year
I'm creating a Django project. I just tried taking the project out of debug,
DEBUG = False
and for some reason all my static files do not show up. They give an error code of 500. How do i fix this?some of settings.py:
DEBUG = True TEMPLATE_DEBUG = DEBUG ... TEMPLATE_LOADERS = ( 'django.template.loaders.filesystem.Loader', 'django.template.loaders.app_directories.Loader', # 'django.template.loaders.eggs.Loader', ) MIDDLEWARE_CLASSES = ( 'django.middleware.common.CommonMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', # 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', )
-
Nachshon Schwartz over 12 yearscan you be more specific, what part? it's pretty long.
-
Timmy O'Mahony over 12 yearsare you serving static files via the django development server or your own webserver. If it's the dev server, where in your URLs are you taking care of the static files? You might find that once you turn off DEBUG, the URLs dealing with serving the static files are disabled
-
Nachshon Schwartz over 12 yearsI am not really sure where I take care of staticfiles, I am using the Django development server. I think that whats happening is what you wrote, the URL's ddealing with staticfiles are disabled upon turning DEBUG off, any idea what i should do?
-
Blairg23 almost 6 yearsHere's an answer from the duplicate question: stackoverflow.com/a/5836728/1224827
-
Blairg23 almost 6 yearsPossible duplicate of Why does DEBUG=False setting make my django Static Files Access fail?
-
Nachshon Schwartz almost 6 years@Blairg23 how can a question from 2011 be a duplicate of question from 2017. Should be the other way around...
-
Blairg23 almost 6 years@Nayish , it says it was asked on April 2011 and yours was June 2011
-
-
Nachshon Schwartz over 12 yearsI'm not sure I understand, is this an answer or a question, where is the part where you say: this is what you should do...?
-
Torsten Engelbrecht over 12 years"You can serve it by appending to your urlpatterns manually or use a server to serve static files (like it is supposed to when running Django projects in non-DEBUG mode)." was the answer? I am adding an example for you if needed, though its the same as serving static files before Django 1.3. And yes, the last one is a questions. So what exception is thrown when it comes to this 500 response? Just interested.
-
Nachshon Schwartz over 12 yearsGreat, that did the trick, I just added that piece of code to my urls.py file and it works like a charm... thanks.
-
MrColes almost 11 yearsMy guess about the 500 is that—since DEBUG is True—django is then trying to render a 404.html template, which raises TemplateDoesNotExist error, so it tries to then render a 500.html template, which also doesn't exist, and just raises a 500. You’d think django would deal with this situation better, but it doesn’t.
-
Torsten Engelbrecht almost 11 yearsNot yet anyway. The coming Django version (1.5) is supposed to handle it better.
-
Caumons over 10 yearsI can confirm that this still works for Django 1.5. Thanks :)
-
The Pied Pipes over 9 yearsthanks. this was all i needed in the end for my issue.
-
Pankaj Anand over 8 yearsI was getting 404 but after adding the url pattern, works fine in apache for Django 1.6.5....
-
Torsten Engelbrecht over 8 years@PankajAnand Its still advised to serve static files via apache directly, rather than through Django. At least once you have production traffic running against your instance it will benefit the performance.
-
Pankaj Anand over 8 years@TorstenEngelbrecht Yes I agree, actually apache level sounds better for performance..
-
Blairg23 almost 6 yearsJust to add in this answer from a duplicate question: stackoverflow.com/a/5836728/1224827