Stop a runnable in a separate Thread
Solution 1
Ok, this is just the very basic threading 101, but let there be another example:
Old-school threading:
class MyTask implements Runnable {
public volatile boolean doTerminate;
public void run() {
while ( ! doTerminate ) {
// do some work, like:
on();
Thread.sleep(1000);
off();
Thread.sleep(1000);
}
}
}
then
MyTask task = new MyTask();
Thread thread = new Thread( task );
thread.start();
// let task run for a while...
task.doTerminate = true;
// wait for task/thread to terminate:
thread.join();
// task and thread finished executing
Edit:
Just stumbled upon this very informative Article about how to stop threads.
Solution 2
Not sure that implementing Executor
is a good idea. I would rather use one of the executors Java provides. They allow you to control your Runnable
instance via Future
interface:
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<?> future = executorService.submit(flashRunnable);
...
future.cancel(true);
Also make sure you free resources that ExecutorService
is consuming by calling executorService.shutdown()
when your program does not need asynchronous execution anymore.
Solution 3
Instead of implementing your own Executor, you should look at ExecutorService. ExecutorService has a shutdown method which:
Initiates an orderly shutdown in which previously submitted tasks are executed, but no new tasks will be accepted.
Solution 4
I would suggest to use the ExecutorService, along with the Furure object, which gives you control over the thread that is being created by the executor. Like the following example
ExecutorService executor = Executors.newSingleThreadExecutor();
Future future = executor.submit(runnabale);
try {
future.get(2, TimeUnit.SECONDS);
} catch (TimeoutException ex) {
Log.warn("Time out expired");
} finally {
if(!future.isDone()&&(!future.isCancelled()))
future.cancel(true);
executor.shutdownNow();
}
This code says that the runnable will be forced to terminate after 2 seconds. Of course, you can handle your Future ojbect as you wish and terminate it according to your requierements
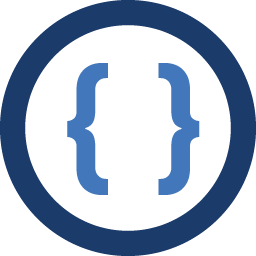
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
Hey there i currently have a problem with my android app. I´m starting an extra thread via implementing the Excecutor Interface:
class Flasher implements Executor { Thread t; public void execute(Runnable r) { t = new Thread(r){ }; t.start(); } }
I start my Runnable like this:
flasherThread.execute(flashRunnable);
but how can i stop it?