store and retrieve javascript arrays into and from HTML5 data attributes
Solution 1
It turned out that you could use the html escaped characters in the element data
attribute to have json-like array (encoded are quotes):
<div id="demo" data-stuff='["some", "string", "here"]'></div>
And then in javascript get it without any additional magic:
var ar = $('#demo').data('stuff');
Check this fiddle out.
Edited (2017)
You don't need to use html escaped characters in the data
attribute.
<div id="demo" data-stuff='["some", "string", "here"]'></div>
Check this new fiddle out.
Solution 2
It depends on what type of data you're storing in the array. If it's just strings (as it appears to be) and you have a character that you know will never be a part of your data (like the comma in my example below) then I would forget about JSON serialization and just use string.split:
<div id="storageElement" data-storeIt="stuff,more stuff"></div>
Then when retrieving:
var storedArray = $("#storageElement").data("storeIt").split(",");
It will handle a bit better than using JSON. It uses less characters and is less "expensive" than JSON.parse
.
But, if you must, your JSON implementation would look something like this:
<div id="storageElement" data-storeIt='["hello","world"]'></div>
And to retrieve:
var storedArray = JSON.parse($("#storageElement").data("storeIt"));
Notice that in this example we had to use semi-quotes ('
) around the data-storeIt
property. This is because the JSON syntax requires us to use quotes around the strings in its data.
Solution 3
The HTML5 data attribute can store only strings, so if you want to store an array you will need to serialize it. JSON will work and it looks like you're on the right path. You just need to use JSON.parse()
once you retrieve the serialized data:
var retrieved_string = $("#storageElement").data('storeit');
var retrieved_array = JSON.parse(retrieved_string);
Reviewing the api documentation, jQuery should try to automatically convert a JSON encoded string provided it is properly encoded. Can you give an example of the value you are storing?
Also note that HTML5 data attribute and jQuery .data()
methods are two distinct things. They interact, but jQuery is more powerful and can store any data type. You could just store a javascript array directly using jQuery without serializing it. But if you need to have it in the markup itself as an HTML5 data attribute, then you are limited only to strings.
Solution 4
For the record, it didn't work with encoded entities for me, but seems that in order to be parsed as an object, the data attribute must be a well formed JSON object.
So I was able to use an object with:
data-myarray="{"key": "value"}"
or maybe just use single quotes:
data-myobject='{"key1": "value1", "key2": value2}'
Time to have fun! :D
Solution 5
You can store any object into node like that:
$('#storageElement').data('my-array', ['a', 'b', 'c']);
var myArray = $('#storageElement').data('my-array');
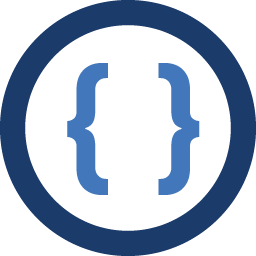
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
How can a javascript
Array
be stored in an HTML5data
attribute?I've tried every variation of
JSON.stringify
cation and escaping characters.What is the precise method to store the array and retrieve it again?
note
I build the array with
[ $("#firstSelectedElement").val(), $("#secondSelectedElement").val() ]
. I retrieveid="storageElement" data-storeIt="stuff"
with$("#storageElement").data('storeit')
.I can never seem to retrieve the data as a true
Array
, only anArray
of characters. -
Grinn about 11 yearsLOL. I hate it when that happens. I guess a trivial answer that makes you go, "duh!" is better than no solution at all though!
-
Grinn about 10 years@Gracchus I just noticed that you said you used my suggestion, but didn't mark it as the answer. Did it end up not working out for you?
-
Vjerci over 9 yearswhat about storring it from html?
-
equivalent8 over 9 yearsFor Rails dudes: The JSON version mentioned here is how HAML is dealing with array setting e.g.:
%button{data: {ids: ['a','b','c']} }
=><button data-ids='["a","b","c"]'>
-
dchhetri over 7 years@Grinn Do you know why
data-storeIt='["hello","world"]'
works butdata-storeIt="['hello','world']"
doesnt? -
Grinn over 7 years@user814628 Although single quotes are cool in JS, they don't fly in JSON. See this answer for more: stackoverflow.com/questions/14355655/…
-
Raff over 7 yearsUse of split(",") is the way to go. One other note: You might want to trim each resulting string element, because if your source has spaces after each comma, those spaces will end up in the array elements. Try this:
$("#storageElement").data("storeIt").split(",").map( e => e.trim() );
-
Grinn over 7 yearsIt's notable that the solution @Raff suggested uses Arrow Functions which aren't supported in IE < Edge. The equivalent would be
...map(function(s) { return s.trim() })
-
Animesh Singh about 7 yearsThanks for the update, It worked. But I don't know why it doesn't works with
data-stuff="['some', 'string', 'here']"
(notice the'
), any guidance would be appreciated. -
Ahmed Mostafa almost 7 yearsThanks a million (Y), this is working fine: '["some", "string", "here"]'
-
nand42 almost 6 years@Animesh Singh : You must use a valid JSON, single quote are not valid JSON.
-
Chad over 5 years@nand42 The single quotes issue was what I was encountering. Works like a charm with double quotes!
-
Henry over 4 yearsBe aware if there is one item in the data it will result in (int) or (string) value. I wrote an answer how to deal with this.
-
Bonifacius Sarumpaet over 3 yearswhat is exactly eval() function in javascript? The only I got online is it executes the argument.
-
Guilherme Muniz over 3 yearseval(string) will execute the string as code. In this code it is taking the string from data attribute and transforming it in array. You can read more about eval function here: developer.mozilla.org/pt-BR/docs/Web/JavaScript/Reference/…
-
Bonifacius Sarumpaet over 3 yearsI see now. Thanks for the brief explanation. But, from what I read online it is dangerous to put eval() on your code due to its vulnerability to be abused by hacker.
-
Ulysse BN over 3 years@BonifaciusSarumpaet you're right, moreover, in most cases eval has really poor performances compared to other solutions (here,
JSON.parse
is much more efficient for instance)