strdup error on g++ with c++0x
Solution 1
-std=gnu++0x (instead of -std=c++0x) does the trick for me; -D_GNU_SOURCE didn't work (I tried with a cross-compiler, but perhaps it works with other kinds of g++).
It appears that the default (no -std=... passed) is "GNU C++" and not "strict standard C++", so the flag for "don't change anything except for upgrading to C++11" is -std=gnu++0x, not -std=c++0x; the latter means "upgrade to C++11 and be stricter than by default".
Solution 2
strdup
may not be included in the library you are linking against (you mentioned mingw). I'm not sure if it's in c++0x or not; I know it's not in earlier versions of C/C++ standards.
It's a very simple function, and you could just include it in your program (though it's not legal to call it simply "strdup" since all names beginning with "str" and a lowercase letter are reserved for implementation extensions.)
char *my_strdup(const char *str) {
size_t len = strlen(str);
char *x = (char *)malloc(len+1); /* 1 for the null terminator */
if(!x) return NULL; /* malloc could not allocate memory */
memcpy(x,str,len+1); /* copy the string into the new buffer */
return x;
}
Solution 3
This page explains that strdup is conforming, among others, to the POSIX and BSD standards, and that GNU extensions implement it. Maybe if you compile your code with "-D_GNU_SOURCE" it works?
EDIT: just to expand a bit, you probably do not need anything else than including cstring on a POSIX system. But you are using GCC on Windows, which is not POSIX, so you need the extra definition to enable strdup.
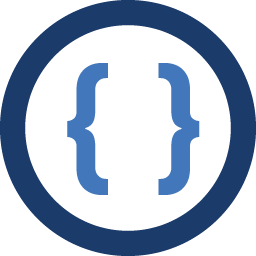
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I have some C++0x code. I was able to reproduce it below. The code below works fine without
-std=c++0x
however i need it for my real code.How do i include strdup in C++0x? with gcc 4.5.2
note i am using mingw. i tried including cstdlib, cstring, string.h and tried using std::. No luck.
>g++ -std=c++0x a.cpp a.cpp: In function 'int main()': a.cpp:4:11: error: 'strdup' was not declared in this scope
code:
#include <string.h> int main() { strdup(""); return 0; }
-
GManNickG about 13 yearsWorks on gcc 4.5.1. Try
#include
<cstring>` and usingstd::strdup
, that's the "C++ way". (Still isn't an answer, though, since that should be valid too, IIRC.) -
Admin about 13 years@GMan: I modified my question. I tried it and no luck :(. As a temp solution i put extern C
_CRTIMP char* __cdecl __MINGW_NOTHROW strdup (const char*) __MINGW_ATTRIB_MALLOC;
in my headers. It works with that.
-
-
Admin about 13 yearsits reserved yet its nonstandard!?
-
Ben over 11 years
-
Random832 over 11 years@Steve That's what I get for coding it as C because it's normally a C function. Yes, it needs a cast in C++.
-
coanor about 11 years
strdup
not in ANSI C,#define
feature test macro may not be the best choice, some otherstrdup-like
may need other feature test (_GNU_SOURCE
,_BSD_SOURCE
, ...). Just implement it. -
einpoklum over 6 yearsThat's an MSVC flag.