String.concat() doesn't do concatenation
37,503
Solution 1
concat
does not alter the invoking strings, but returns a new one.
You may assign each resulting String
to your result like this.-
messages = messages.concat(String.valueOf(random));
messages = messages.concat(" ");
messages = messages.concat(String.valueOf(ch));
messages = messages.concat(" ");
Or just use the overloaded operator +
messages = String.valueOf(random) + " " + String.valueOf(ch) + " ";
Solution 2
Strings
are immutable. You can either append the result using String
concatenation as shown in other answers or you can use StringBuilder
StringBuilder messages = new StringBuilder();
messages.append(String.valueOf(random));
messages.append(" ");
messages.append(String.valueOf(ch));
messages.append(" ");
Have a look at How do I concatenate two strings in Java?
Edit: (to insert at beginning of String
)
messages.insert(0, "newstring");
Solution 3
A better way is :
Random r = new Random();
int random = r.nextInt(1000);
StringBuilder str = new StringBuilder(String.valueOf(random));
str.append("s")
str.append(String.valueOf(random))
str.append(" ");
str.append(String.valueOf(ch));
Solution 4
messages += String.valueOf(random) + " " + String.valueOf(ch) + " ";
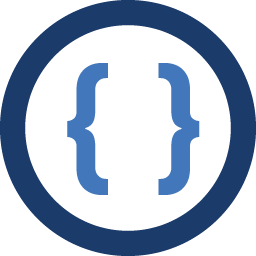
Author by
Admin
Updated on July 18, 2020Comments
-
Admin almost 4 years
I am trying to concatenate a String with an int and char including spaces, but not getting any concatenation. What is the reason?
private String messages; Random r = new Random(); int random = r.nextInt(1000); char ch='s'; messages.concat(String.valueOf(random)); messages.concat(" "); messages.concat(String.valueOf(ch)); messages.concat(" ");
-
Admin over 10 yearshow can I concat any specified string at the beginning of the string?
-
ssantos over 10 yearsWould require to initialize
messages
to "" in its declaration -
Cruncher over 10 yearsYes, this assumes that messages is initialized. The question was about concatenating.
-
Cruncher over 10 years@Martin
messages = "newstring" + messages
-
Sotirios Delimanolis over 10 years@Martin Call it the other way around.
"yourString".concat(message);
if you want to use a method call. -
ssantos over 10 yearsUsing the
+
operator again, something likeresultingString = specifiedString + originalString
-
Admin over 10 yearsis there any way by which i can append any specified string at the beginning of the string?
-
Ahmed Adel Ismail over 10 yearsthats better yes, since you wont need to create all those string Objects throw concat() method
-
jmaculate almost 10 yearsThis should be the accepted answer. StringBuilder doesn't alloc a new string for every addition. Any time you're concat'ing more than 2 strings it's the way to go
-
Jay about 8 yearsNote: the '+' operator usage will actually use a StringBuilder
-
Jay about 8 yearsString.valueOf is really not needed, something like: "" + random + " " + ch + " "
-
Reimeus over 6 yearsyes,
StringBuilder
has an insert method with an offset position