String convert to Int and replace comma to Plus sign
Solution 1
- Use
components(separatedBy:)
to break up the comma-separated string. - Use
trimmingCharacters(in:)
to remove spaces before and after each element - Use
Int()
to convert each element into an integer. - Use
compactMap
(previously calledflatMap
) to remove any items that couldn't be converted toInt
. Use
reduce
to sum up the array ofInt
.let input = " 98 ,99 , 97, 96 " let values = input.components(separatedBy: ",").compactMap { Int($0.trimmingCharacters(in: .whitespaces)) } let sum = values.reduce(0, +) print(sum) // 390
Solution 2
For Swift 3 and Swift 4.
Simple way: Hard coded. Only useful if you know the exact amount of integers coming up, wanting to get calculated and printed/used further on.
let string98: String = "98"
let string99: String = "99"
let string100: String = "100"
let string101: String = "101"
let int98: Int = Int(string98)!
let int99: Int = Int(string99)!
let int100: Int = Int(string100)!
let int101: Int = Int(string101)!
// optional chaining (if or guard) instead of "!" recommended. therefore option b is better
let finalInt: Int = int98 + int99 + int100 + int101
print(finalInt) // prints Optional(398) (optional)
Fancy way as a function: Generic way. Here you can put as many strings in as you need in the end. You could, for example, gather all the strings first and then use the array to have them calculated.
func getCalculatedIntegerFrom(strings: [String]) -> Int {
var result = Int()
for element in strings {
guard let int = Int(element) else {
break // or return nil
// break instead of return, returns Integer of all
// the values it was able to turn into Integer
// so even if there is a String f.e. "123S", it would
// still return an Integer instead of nil
// if you want to use return, you have to set "-> Int?" as optional
}
result = result + int
}
return result
}
let arrayOfStrings = ["98", "99", "100", "101"]
let result = getCalculatedIntegerFrom(strings: arrayOfStrings)
print(result) // prints 398 (non-optional)
Solution 3
let myString = "556"
let myInt = Int(myString)
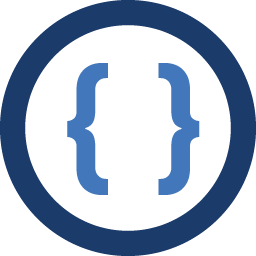
Admin
Updated on September 24, 2020Comments
-
Admin over 3 years
Using Swift, I'm trying to take a list of numbers input in a text view in an app and create a sum of this list by extracting each number for a grade calculator. Also the amount of values put in by the user changes each time. An example is shown below:
String of: 98,99,97,96... Trying to get: 98+99+97+96...
Please Help! Thanks