String.replace result is ignored?
Solution 1
String
objects are immutable.
From the docs:
public String replace(char oldChar,char newChar)
Returns a string resulting from replacing all occurrences of oldChar in this string with newChar.
Hope this helps.
Solution 2
IntelliJ is complaining that you're calling a method whose only effect is to return a value (String.replace) but you're ignoring that value. The program isn't doing anything at the moment because you're throwing away all the work it does.
You need to use the return value.
There are other bugs in there too. You might be able to progress a little further if you use some of this code:
StringBuilder convertedPhoneNumber = new StringBuilder();
// Your loop begins here
char curCharacter = phoneNumber.charAt(i);
if (curCharacter == 'a') {
convertedPhoneNumber.append("2");
}
// More conditional logic and rest of loop goes here.
return convertedPhoneNumber.toString();
Solution 3
I had the same problem, but i did it like this:
String newWord = oldWord.replace(oldChar,newChar);
Solution 4
use that statement
string = string.replace(string.charAt(i));
Why? String is an immutable object. Look at this thread to get a complete explanation. This a fundemental part of Java, so make sure you learn it well.
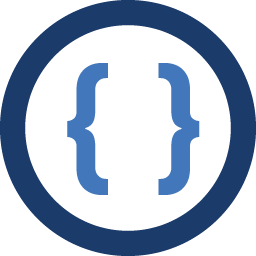
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
So i'm using IntelliJ and the replace buzzword is highlighted. Most of the tips are over my head so i ignore them, but what i got for this one was that the result of string.replace is ignored. Why?
would i need something like (
string = string.replace(string.charAt(i));
)?import java.util.Scanner; public class PhoneNumberDecipher { public static String phoneNumber; public static String Decipher(String string) { string = phoneNumber; for(int i =0; i<=phoneNumber.length(); i++) { if(string.equalsIgnoreCase("A") ||string.equalsIgnoreCase("B") ||string.equalsIgnoreCase("C")) { string.replace(string.charAt(i),'2') } else if(string.equalsIgnoreCase("D") ||string.equalsIgnoreCase("E") ||string.equalsIgnoreCase("F")) { string.replace(string.charAt(i),'3'); } else if(string.equalsIgnoreCase("G") ||string.equalsIgnoreCase("H") ||string.equalsIgnoreCase("I")) { string.replace(string.charAt(i),'4'); } else if(string.equalsIgnoreCase("J") ||string.equalsIgnoreCase("K") ||string.equalsIgnoreCase("L")) { string.replace(string.charAt(i),'5'); } else if(string.equalsIgnoreCase("M") ||string.equalsIgnoreCase("N") ||string.equalsIgnoreCase("O")) { string.replace(string.charAt(i),'6'); } else if(string.equalsIgnoreCase("P") ||string.equalsIgnoreCase("Q") ||string.equalsIgnoreCase("R") || string.equalsIgnoreCase("S")) { string.replace(string.charAt(i),'7'); } else if(string.equalsIgnoreCase("T") ||string.equalsIgnoreCase("U") ||string.equalsIgnoreCase("V")) { string.replace(string.charAt(i),'8'); } else if(string.equalsIgnoreCase("W") ||string.equalsIgnoreCase("X") ||string.equalsIgnoreCase("Y") ||string.equalsIgnoreCase("Z")) { string.replace(string.charAt(i),'9'); } } return string; } public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Please Enter a Phone Number you Wish to Decipher..."); phoneNumber = input.nextLine(); System.out.print(Decipher(phoneNumber)); } }