String representation of time_t?
Solution 1
Try std::stringstream
.
#include <string>
#include <sstream>
std::stringstream ss;
ss << seconds;
std::string ts = ss.str();
A nice wrapper around the above technique is Boost's lexical_cast
:
#include <boost/lexical_cast.hpp>
#include <string>
std::string ts = boost::lexical_cast<std::string>(seconds);
And for questions like this, I'm fond of linking The String Formatters of Manor Farm by Herb Sutter.
UPDATE:
With C++11, use to_string()
.
Solution 2
Try this if you want to have the time in a readable string:
#include <ctime>
std::time_t now = std::time(NULL);
std::tm * ptm = std::localtime(&now);
char buffer[32];
// Format: Mo, 15.06.2009 20:20:00
std::strftime(buffer, 32, "%a, %d.%m.%Y %H:%M:%S", ptm);
For further reference of strftime() check out cppreference.com
Solution 3
The top answer here does not work for me.
See the following examples demonstrating both the stringstream and lexical_cast answers as suggested:
#include <iostream>
#include <sstream>
int main(int argc, char** argv){
const char *time_details = "2017-01-27 06:35:12";
struct tm tm;
strptime(time_details, "%Y-%m-%d %H:%M:%S", &tm);
time_t t = mktime(&tm);
std::stringstream stream;
stream << t;
std::cout << t << "/" << stream.str() << std::endl;
}
Output: 1485498912/1485498912 Found here
#include <boost/lexical_cast.hpp>
#include <string>
int main(){
const char *time_details = "2017-01-27 06:35:12";
struct tm tm;
strptime(time_details, "%Y-%m-%d %H:%M:%S", &tm);
time_t t = mktime(&tm);
std::string ts = boost::lexical_cast<std::string>(t);
std::cout << t << "/" << ts << std::endl;
return 0;
}
Output: 1485498912/1485498912 Found: here
The 2nd highest rated solution works locally:
#include <iostream>
#include <string>
#include <ctime>
int main(){
const char *time_details = "2017-01-27 06:35:12";
struct tm tm;
strptime(time_details, "%Y-%m-%d %H:%M:%S", &tm);
time_t t = mktime(&tm);
std::tm * ptm = std::localtime(&t);
char buffer[32];
std::strftime(buffer, 32, "%Y-%m-%d %H:%M:%S", ptm);
std::cout << t << "/" << buffer;
}
Output: 1485498912/2017-01-27 06:35:12 Found: here
Solution 4
the function "ctime()" will convert a time to a string. If you want to control the way its printed, use "strftime". However, strftime() takes an argument of "struct tm". Use "localtime()" to convert the time_t 32 bit integer to a struct tm.
Solution 5
The C++ way is to use stringstream.
The C way is to use snprintf() to format the number:
char buf[16];
snprintf(buf, 16, "%lu", time(NULL));
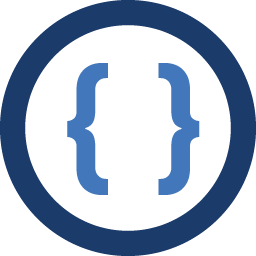
Admin
Updated on February 19, 2020Comments
-
Admin about 4 years
time_t seconds; time(&seconds); cout << seconds << endl;
This gives me a timestamp. How can I get that epoch date into a string?
std::string s = seconds;
does not work
-
Admin almost 15 yearsnulldevice, I wasn't clear above, but I wanted a string representation of the epoch date (timestamp).
-
nitzanms about 7 yearstime_t isn't necessarily unsigned or of the same size as a long. In fact, it is usually signed: stackoverflow.com/questions/471248/…
-
Lightness Races in Orbit about 5 yearsI don't get it. Your first two snippets show that the stringisation worked perfectly.
-
Lightness Races in Orbit about 5 yearsPossibly not the best function name, and your code could do with some documenting comments ... but otherwise this isn't terrible ;)
-
No Refunds No Returns about 5 yearsI'd probably do a lot of things differently if do-overs were a real thing. All I can really say is appreciate my .net's .ToString("yyyy-MM-dd HH:mm:ss.fffffff") method more today than I did yesterday.