Styled-components: Override component style inside a new component
There are a couple of issues to address.
You can follow along on CodeSandbox.
1. Export Header
component from Component A
You need to make Header
component available outside Component A
so that it can be referenced within Component B
.
import React from "react";
import styled from "styled-components";
export const Header = styled.h2`
padding-left: 0;
`;
export default ({ className = "", title }) => (
<div className={className}>
<Header>{title}</Header>
</div>
);
2. Errors in Component B
There are three issues here.
- You need to pass the component name, not the instance to
styled()
function.
Instead of const CustomA = styled(<A />)
where <A />
is an instance,
Do const CustomA = styled(A)
.
-
You need to import
Header
component exported fromComponent A
.
Now you can reference is withinstyled(A)
as ${Header}.import styled from "styled-components"; import A, { Header } from "./CustomA"; const CustomA = styled(A)` ${Header} { padding-left: 20px; } `; export default () => <CustomA title="Component B Content" />;
The last issue is that, you aren't passing the
title
(I also didclassName = ""
inComponent A
to make it optional).
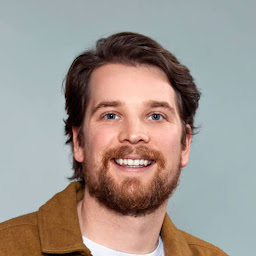
Henrik Fischer Bjelland
Updated on June 29, 2022Comments
-
Henrik Fischer Bjelland almost 2 years
I am trying to override the style of a component inside another component.
So, I have a component A, with some div's inside(Wrapper, Header). In a new component, I am trying to override component A. Inside that override I want some new styling to the Header component. I know I can reference a component inside the same component but I can't find any info about referencing inside a new component.
// Component A import React from "react"; export default ({ className, title }) => ( <Wrapper className={className}> <Header>{title}</Header> </Wrapper> ) ); const Header = styled.h2` padding-left: 0; `; // Component B import React from "react"; export default () => ( <CustomA> /* content */ </CustomA> ) ); const CustomA = styled(<A />)` ${Header} { padding-left: 20px; } `;
I expect Header to be changed but I get "Header is not defined".
-
nircraft almost 5 yearsWhile this code snippet may be the solution, including an explanation really helps to improve the quality of your post. Remember that you are answering the question for readers in the future, and those people might not know the reasons for your code suggestion.
-
Henrik Fischer Bjelland almost 5 yearsThanks! This solved it! I was missing the import of { Header }.
-
dance2die almost 5 years@HenrikFischerBjelland Glad it worked 😀. Would you mark it as an answer to others can find the answer & there is no more replies needed?