Swift 4 decodable json arrays
17,324
Solution 1
Please check below :
I din't add for all the keys. I added for some in attributes
.
struct AnimeJsonStuff: Decodable {
let data: [AnimeDataArray]
}
struct AnimeLinks: Codable {
var selfStr : String?
private enum CodingKeys : String, CodingKey {
case selfStr = "self"
}
}
struct AnimeAttributes: Codable {
var createdAt : String?
private enum CodingKeys : String, CodingKey {
case createdAt = "createdAt"
}
}
struct AnimeRelationships: Codable {
var links : AnimeRelationshipsLinks?
private enum CodingKeys : String, CodingKey {
case links = "links"
}
}
struct AnimeRelationshipsLinks: Codable {
var selfStr : String?
var related : String?
private enum CodingKeys : String, CodingKey {
case selfStr = "self"
case related = "related"
}
}
struct AnimeDataArray: Codable {
let id: String?
let type: String?
let links: AnimeLinks?
let attributes: AnimeAttributes?
let relationships: [String: AnimeRelationships]?
private enum CodingKeys: String, CodingKey {
case id = "id"
case type = "type"
case links = "links"
case attributes = "attributes"
case relationships = "relationships"
}
}
Json parsing :
func jsonDecoding() {
let jsonUrlString = "https://kitsu.io/api/edge/anime"
guard let url = URL(string: jsonUrlString) else {return} //
URLSession.shared.dataTask(with: url) { (data, response, err) in
guard let data = data else {return}
do {
let animeJsonStuff = try JSONDecoder().decode(AnimeJsonStuff.self, from: data)
for anime in animeJsonStuff.data {
print(anime.id)
print(anime.type)
print(anime.links?.selfStr)
print(anime.attributes?.createdAt)
for (key, value) in anime.relationships! {
print(key)
print(value.links?.selfStr)
print(value.links?.related)
}
}
} catch let jsonErr {
print("Error serializing json", jsonErr)
}
}.resume()
}
Solution 2
Answer for title "Swift 4 decodable json arrays"
let decoder = JSONDecoder()
do {
let array = try decoder.decode([YouCodableStruct].self, from: response.data!)
debugPrint(array)
} catch {
debugPrint("Error occurred")
}
http://andrewmarinov.com/parsing-json-swift-4/
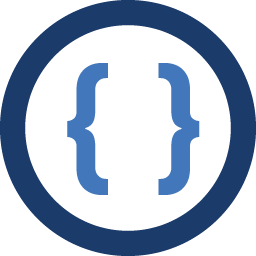
Author by
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
So I am currently woking on a program to parse a JSON Api which is linked at the bottom
When I run the code I get some output but not all
Mainly for the optional type of Anime which is good because it shows that it works but I also want to access the name and release date and languages however I have no idea how to work with JSON arrays like this in swift 4. I will attach my current code below.
import UIKit struct AnimeJsonStuff: Decodable { let data: [AnimeDataArray] } struct AnimeDataArray: Decodable { let type: String? } class OsuHomeController: UICollectionViewController, UICollectionViewDelegateFlowLayout { func jsonDecoding() { let jsonUrlString = "https://kitsu.io/api/edge/anime" guard let url = URL(string: jsonUrlString) else {return} // URLSession.shared.dataTask(with: url) { (data, response, err) in guard let data = data else {return} do { let animeJsonStuff = try JSONDecoder().decode(AnimeJsonStuff.self, from: data) print(animeJsonStuff.data) let animeDataArray = try JSONDecoder().decode(AnimeDataArray.self, from: data) print(animeDataArray.type as Any) } catch let jsonErr { print("Error serializing json", jsonErr) } }.resume() } }
I have more code after that but it's just for setting up custom collectionViewCells.
Also here is the link to the api