Swift - could not dequeue a view of kind: UICollectionElementKindCell
Solution 1
It is because you haven't registered your xib to UICollectionView
. If you are using UICollectionViewCell
from Xib, you must register it first.
In viewDidLoad:
write it:
if let xib = NSNib.init(nibNamed: "TemplateNBgCollectionItem", bundle: nil) {
self.collectionView.register(xib, forItemWithIdentifier: NSUserInterfaceItemIdentifier(rawValue: "cvItem"))
}
Solution 2
In viewDidLoad
method:
You must register your custom cell class/xib name with collectionView object.
If you have only class custom class then you register by following way (No xib file)
collectionView.register(CustomCell.self, forCellWithReuseIdentifier: "cellId")
If you have both class as well as xib file then you can register by this way
collectionView.register(UINib(nibName: "CustomCell", bundle: nil), forCellWithReuseIdentifier: "cellId")
Solution 3
From the code snippets you pasted, I don't see you register the cell before you want to dequeue/use it. Need to register the cell before use as the error described.
tableView.register(UITableViewCell.self, forCellReuseIdentifier: "DefaultCell")
For your quick reference, please see below post - https://www.hackingwithswift.com/example-code/uikit/how-to-register-a-cell-for-uitableviewcell-reuse
Edit after screenshot posted(Please accept this answer if works for you ^_^)
From the screenshot, you are using a custom cell class 'CustomCell', after you set the correct identifier, please make sure setup the correct class name as CustomCell in the identity inspector of right panel. Refer to below screenshot.
Solution 4
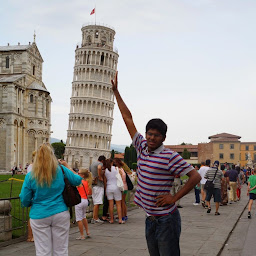
Sid
Updated on July 09, 2022Comments
-
Sid almost 2 years
I got this error message when trying to load my project
Terminating app due to uncaught exception 'NSInternalInconsistencyException', reason: 'could not dequeue a view of kind: UICollectionElementKindCell with identifier CustomCell - must register a nib or a class for the identifier or connect a prototype cell in a storyboard'
My code is:
extension ViewController: JTAppleCalendarViewDelegate, JTAppleCalendarViewDataSource { func configureCalendar(_ calendar: JTAppleCalendarView) -> ConfigurationParameters { formatter.dateFormat = "yyyy MM dd" formatter.timeZone = Calendar.current.timeZone formatter.locale = Calendar.current.locale let startDate = formatter.date(from: "2017 01 01")! let endDate = formatter.date(from: "2017 12 31")! let parameters = ConfigurationParameters(startDate: startDate, endDate: endDate) return parameters } func calendar(_ calendar: JTAppleCalendarView, cellForItemAt date: Date, cellState: CellState, indexPath: IndexPath) -> JTAppleCell { let cell = calendar.dequeueReusableJTAppleCell(withReuseIdentifier: "CustomCell", for: indexPath) as! CustomCell cell.dateLabel.text = cellState.text return cell } }
Please help me debug this problem. Thank you.
Edit: I have added the identifier for the cell, but still the error exists.
-
Sid about 7 yearsI am sorry, but I am very new to this. When i tried your suggestion, I get the following error - Use of unresolved identifier 'collectionView'
-
ankit about 7 yearsoh thats because I have taken collectionView as dummy text. what you have to do is this: make an IBOutlet of UICollectionView named collectionView. Then you are good to go
-
Sid about 7 yearsCan you please tell me what exactly I need to do and where i need to put it. Sorry, but this is my first time doing all of this
-
ankit about 7 yearsGo to that controller on IB. Ctrl + drag to your this controller class. then when it prompts , use name : collectionView
-
Aaron about 7 yearsI see your screenshot after you update, just be careful when you connect the IBOutlet, and make sure the name is matched with your xib. or you can re-connect it to double check. Good Luck
-
Saeed Rahmatolahi almost 7 yearsI used This But I still having problem with line let cell = calendar.dequeueReusableJTAppleDayCellView(withReuseIdentifier: "JTCustomCell", for: indexPath) as! JTCustomCell