Swift Detect Touch Anywhere
Solution 1
Dismissing a modal view turns out to be surprisingly easy. All you need to do is call dismissViewControllerAnimated(true, completion: nil)
. Thus, to do what I wanted, all I needed to do was this:
override func touchesEnded(touches: NSSet, withEvent event: UIEvent)
{
dismissViewControllerAnimated(true, completion: nil)
super.touchesEnded(touches, withEvent: event)
}
Solution 2
Swift 3.0
// Add this to your UIViewController class
override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) {
//Do thing here
}
Solution 3
I found a great way of doing this by adding a UIGestureRecognizer.
In AppDelegate conform to UIGestureRecognizerDelegate, by adding it to your
class AppDelegate: UIResponder, UIApplicationDelegate, UIGestureRecognizerDelegate {
...
Add tapGestures
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
// add tapGestures to entire application
let tapGesture = UITapGestureRecognizer(target: self, action: nil)
tapGesture.delegate = self
window?.addGestureRecognizer(tapGesture)
return true
}
add the gestureRecognizer protocol function:
func gestureRecognizer(_ gestureRecognizer: UIGestureRecognizer, shouldReceive touch: UITouch) -> Bool {
// User tapped on screen, do something
return false
}
Solution 4
I personally do not know how to dismiss popover's since I haven't used them, but I can fix one of your problems. You say that you want to dismiss a popover or modal when the user touches anywhere on the screen. Here is a function that triggers when the screen is touched in any location, you can read more about it in the apple documents.
override func touchesBegan(touches: NSSet, withEvent event: UIEvent) {
}
Solution 5
Swift 4.2 :
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
// Touch began
}
override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) {
// Touch end
}
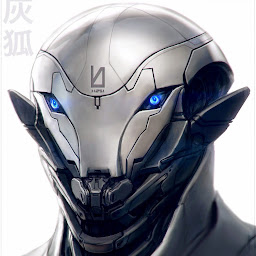
7OO Tnega Terces
Updated on July 04, 2022Comments
-
7OO Tnega Terces almost 2 years
I'm writing my first app, using Swift, and I need a popover or modal view that can be dismissed by touching anywhere on the screen.
I'm writing an IOU app and am currently working on the view where the user enters the lenders and how much they lend. Obviously each lender needs to have a unique name, and I'd like a popover or modal view to appear whenever the user tries to enter the same name twice asking them to change the name. To lessen the irritation factor, I'd like to make it so that the user can tap anywhere on the screen to dismiss the warning, rather than on a specific button.
I found this answer: Detect touch globally, and I think it might work for me, but I know nothing of Objective-C, just Swift, and couldn't understand enough to know what to do.
-
7OO Tnega Terces over 9 yearsThanks! This is just the function I wanted!