Syntax sugar: _* for treating Seq as method parameters
Solution 1
Generally, the :
notation is used for type ascription, forcing the compiler to see a value as some particular type. This is not quite the same as casting.
val b = 1 : Byte
val f = 1 : Float
val d = 1 : Double
In this case, you're ascribing the special varargs type. This mirrors the asterisk notation used for declaring a varargs parameter and can be used on a variable of any type that subclasses Seq[T]
:
def f(args: String*) = ... //varargs parameter, use as an Array[String]
val list = List("a", "b", "c")
f(list : _*)
Solution 2
That's scala syntax for exploding an array. Some functions take a variable number of arguments and to pass in an array you need to append : _*
to the array argument.
Solution 3
Variable (number of) Arguments are defined using *. For example,
def wordcount(words: String*) = println(words.size)
wordcount expects a string as parameter,
scala> wordcount("I")
1
but accepts more Strings as its input parameter (_* is needed for Type Ascription)
scala> val wordList = List("I", "love", "Scala")
scala> wordcount(wordList: _*)
3
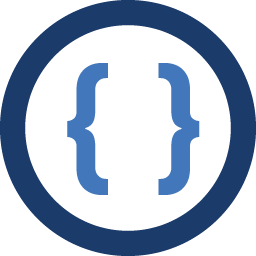
Admin
Updated on January 19, 2020Comments
-
Admin over 4 years
I just noticed this construct somewhere on web:
val list = List(someCollection: _*)
What does
_*
mean? Is this a syntax sugar for some method call? What constraints should my custom class satisfy so that it can take advantage of this syntax sugar? -
retronym over 13 yearsNitpick: the argument need only be a
Seq
, or implicitly convertible to aSeq
. -
gursahib.singh.sahni almost 7 yearsi tried returning an array of String like
String*
, i get an error that cannot resolve * . If i returnArray[String]
and pass it to a method with args(args: String*)
it says expecting String and notArray[String]