System.Text.Encoding.GetEncoding("iso-8859-1") throws PlatformNotSupportedException?
Solution 1
I would try to use "windows-1252" as encoding string. According to Wikipedia, Windows-1252 is a superset of ISO-8859-1.
System.Text.Encoding.GetEncoding(1252)
Solution 2
This MSDN article says:
The .NET Compact Framework supports character encoding on all devices: Unicode (BE and LE), UTF8, UTF7, and ASCII.
There is limited support for code page encoding and only if the encoding is recognized by the operating system of the device.
The .NET Compact Framework throws a PlatformNotSupportedException if the a required encoding is not available on the device.
I believe all (or at least many) of the ISO encodings are code-page encodings and fall under the "limited support" rule. UTF8 is probably your best bet as a replacement.
Solution 3
I know its a bit later but I made an implementation for .net cf of encoding ISO-8859-1, I hope this could help:
namespace System.Text
{
public class Latin1Encoding : Encoding
{
private readonly string m_specialCharset = (char) 0xA0 + @"¡¢£¤¥¦§¨©ª«¬®¯°±²³´µ¶·¸¹º»¼½¾¿ÀÁÂÃÄÅÆÇÈÉÊËÌÍÎÏÐÑÒÓÔÕÖרÙÚÛÜÝÞßàáâãäåæçèéêëìíîïðñòóôõö÷øùúûüýþÿ";
public override string WebName
{
get { return @"ISO-8859-1"; }
}
public override int CodePage
{
get { return 28591; }
}
public override int GetByteCount(char[] chars, int index, int count)
{
return count;
}
public override int GetBytes(char[] chars, int charIndex, int charCount, byte[] bytes, int byteIndex)
{
if (chars == null)
throw new ArgumentNullException(@"chars", @"null array");
if (bytes == null)
throw new ArgumentNullException(@"bytes", @"null array");
if (charIndex < 0)
throw new ArgumentOutOfRangeException(@"charIndex");
if (charCount < 0)
throw new ArgumentOutOfRangeException(@"charCount");
if (chars.Length - charIndex < charCount)
throw new ArgumentOutOfRangeException(@"chars");
if (byteIndex < 0 || byteIndex > bytes.Length)
throw new ArgumentOutOfRangeException(@"byteIndex");
for (int i = 0; i < charCount; i++)
{
char ch = chars[charIndex + i];
int chVal = ch;
bytes[byteIndex + i] = chVal < 160 ? (byte)ch : (chVal <= byte.MaxValue ? (byte)m_specialCharset[chVal - 160] : (byte)63);
}
return charCount;
}
public override int GetCharCount(byte[] bytes, int index, int count)
{
return count;
}
public override int GetChars(byte[] bytes, int byteIndex, int byteCount, char[] chars, int charIndex)
{
if (chars == null)
throw new ArgumentNullException(@"chars", @"null array");
if (bytes == null)
throw new ArgumentNullException(@"bytes", @"null array");
if (byteIndex < 0)
throw new ArgumentOutOfRangeException(@"byteIndex");
if (byteCount < 0)
throw new ArgumentOutOfRangeException(@"byteCount");
if (bytes.Length - byteIndex < byteCount)
throw new ArgumentOutOfRangeException(@"bytes");
if (charIndex < 0 || charIndex > chars.Length)
throw new ArgumentOutOfRangeException(@"charIndex");
for (int i = 0; i < byteCount; ++i)
{
byte b = bytes[byteIndex + i];
chars[charIndex + i] = b < 160 ? (char)b : m_specialCharset[b - 160];
}
return byteCount;
}
public override int GetMaxByteCount(int charCount)
{
return charCount;
}
public override int GetMaxCharCount(int byteCount)
{
return byteCount;
}
}
}
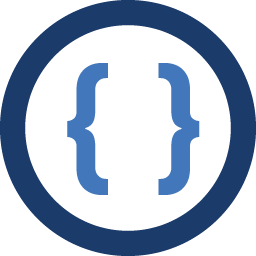
Admin
Updated on July 16, 2022Comments
-
Admin almost 2 years
See subject, note that this question only applies to the .NET compact framework. This happens on the emulators that ship with Windows Mobile 6 Professional SDK as well as on my English HTC Touch Pro (all .NET CF 3.5). iso-8859-1 stands for Western European (ISO), which is probably the most important encoding besides us-ascii (at least when one goes by the number of usenet posts).
I'm having a hard time to understand why this encoding is not supported, while the following ones are supported (again on both the emulators & my HTC):
- iso-8859-2 (Central European (ISO))
- iso-8859-3 (Latin 3 (ISO))
- iso-8859-4 (Baltic (ISO))
- iso-8859-5 (Cyrillic (ISO))
- iso-8859-7 (Greek (ISO))
So, is support for say Greek more important than support for German, French and Spanish? Can anyone shed some light on this?
Thanks!
Andreas
-
Admin over 15 yearsHi It seems none of the linked pages contain information that could answer the question "Why is ISO-8859-7 supported while ISO-8859-1 isn't?"
-
Admin over 15 yearsHi This approach is very cumbersome in some situations, e.g. when you have an XML stream with a ISO-8859-1 encoding. You'd need to replace the encoding "iso-8859-1" with "windows-1252" before feeding it to the XML reader.
-
Otis over 15 yearsI thought the "limited support for code page supporting" wrapped it up rather succinctly, myself. I believe you're stuck with using UTF8.
-
Admin over 15 yearsI don't see how UTF-8 "contains" iso-8859-1. In the former, only pure ASCII characters can be encoded in one byte, in the latter also chars like e.g. Ä can be encoded in one byte. So if you have a file encoded in ISO-8859-1 you cannot correctly read it with the UTF-8 encoding.
-
ctacke over 15 yearsAh, I see - you have text already encoded. I was thinking that you simply needed a mechanism for encoding all of these chanracters