Tabs' position of JTabbedPane
If your target platform is Mac, you would most likely follow the style guides for OSX. Java Development Guide for Mac. Page 40 talks about Tabbed Panes.
By default you can only position the tabs on the different sides, for example:
// Alternative placements:
// JTabbedPane.TOP
// JTabbedPane.RIGHT
// JTabbedPane.LEFT
// JTabbedPane.BOTTOM.
tabbedPane.setTabPlacement(JTabbedPane.TOP);
But if you want to have the tabs on top, but adjusted to left rather than center, you need to either pick a different look and feel, or modify the system look and feel. This is possible but a bit more involved, and as pointed out allready, it is not reccomended. You have to perform all changes before you display anything. For a starting point on that topic, refer to How to write a Custom Look and Feel
For running example see code:
import java.awt.*;
import javax.swing.*;
public class Tab {
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame("TabbedPane");
frame.getContentPane().add(new TabView());
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setMinimumSize(new Dimension(800, 450));
frame.setLocationRelativeTo(null); // Center
frame.pack();
frame.setVisible(true);
}
});
}
static class TabView extends JPanel {
private JTabbedPane tabbedPane;
TabView() {
createComponents();
makeLayout();
}
private void createComponents() {
tabbedPane = new JTabbedPane();
tabbedPane.addTab("Hello", new JLabel("World"));
tabbedPane.addTab("Goodbye", new JLabel("Sunshine"));
}
private void makeLayout() {
setLayout(new BorderLayout());
// Alternative placements: JTabbedPane.TOP, JTabbedPane.RIGHT, JTabbedPane.LEFT JTabbedPane.BOTTOM.
tabbedPane.setTabPlacement(JTabbedPane.TOP);
// Alternative layout policies: JTabbedPane.WRAP_TAB_LAYOUT or JTabbedPane.SCROLL_TAB_LAYOUT.
tabbedPane.setTabLayoutPolicy(JTabbedPane.WRAP_TAB_LAYOUT);
add(tabbedPane, BorderLayout.CENTER);
}
}
}
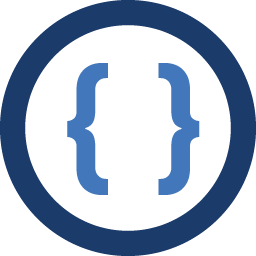
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I would like to know how can I set the tabs' position of JTabbedPane starting from the left.By default they are in a central position because i use Mac OS X.
If you need more info please let me know.
see this image to get an idea of what I get in my mac using the default JTabbedPane:
-
Abin about 11 yearsIts not working in MAC, 2 tabs are at the center.. Can u help me plsss
-
Skjalg about 11 yearsIf you have 2 tabs in the middle, it is indeed working. As pointed out in the above answer, if you want something else, you should switch look and feel of your application, or you can write a Custom Look and Feel. Before you start however, you should ask yourself "why?". Is it because you do not like the Apple Look and Feel? Is it because someone asked you to do it? If yes, then you should ask them "why?". Most likely you will find the answer you should be looking for. See also en.wikipedia.org/wiki/5_Whys