Tap on UITextField's clear button hides keyboard instead of clearing text
Solution 1
Just clear the field, resignFirstResponder
(if you want to hide keyboard) and return NO
/false
Note: set Attributes inspector property of UITextField
Clear Button -> Appears while editing
so it will display the clear button while editing in the text field.
// Objective-C
-(BOOL)textFieldShouldClear:(UITextField *)textField
{
textField.text = @"";
[textField resignFirstResponder];
return NO;
}
// Swift
func textFieldShouldClear(textField: UITextField) -> Bool {
textField.text = ""
textField.resignFirstResponder()
return false
}
Solution 2
Try this code after you attach delegate of uitextifield
-(BOOL)textFieldShouldClear:(UITextField *)textField
{
return true;
}
Solution 3
First, check all the code blocks that related to your UITextField
(especially the code yourTextField.hidden = YES;
)
Put break points and analyze every UITextField
delegates that you implemented.
(textFieldDidEndEditing
,textFieldShouldEndEditing
,textFieldShouldReturn.etc
.)
OR
Implement the textFieldShouldClear
delegate and write the code here to visible and clear your UITextField
To do this, you have to set the clearButtonMode
as below,
yourTextField.clearButtonMode = UITextFieldViewModeWhileEditing;
yourTextField.delegate = self;
//For active keyboard again
[yourTextField becomeFirstResponder];
Then implement the textFieldShouldClear
delegate
YourClass.h
@interface className : UIViewController <UITextFieldDelegate>
YourClass.m
-(BOOL)textFieldShouldClear:(UITextField *)textField {
yourTextField.hidden = NO;
yourTextField.text = @"";
return YES;
}
Solution 4
Just make sure U've given these two
editingTextField.delegate = self;
editingTextField.clearButtonMode = UITextFieldViewModeWhileEditing;
TextFieldShouldClear is needed only if you need to do some customizations :-)
Are you doing some thing in this method?
Maybe you are are calling resignFirstResponder in this delegate method, thats why the keyboard is getting dismissed.
Please go through the delegate methods, and check what u r doing exactly.
Solution 5
This issue happened also if you have
yourTextField.clearButtonMode = UITextFieldViewModeNever;
Check this line and delete it or change view mode..
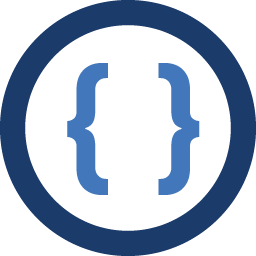
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
In iPhone, I have a view which has a
UITextField
. When I tap on the clear button ofUITextField
's the keyboard dismissed instead of clearing the text in theUITextField
. On an iPad it is working correctly. What can I do to fix this? -
Admin almost 12 yearsthanks for all your answers I got the problem where I'm making mistake.Now I'm done correctly