Tensorflow Data Adapter Error: ValueError: Failed to find data adapter that can handle input
Solution 1
Have you checked whether your training/testing data and training/testing labels are all numpy arrays? It might be that you're mixing numpy arrays with lists.
Solution 2
You can avoid this error by converting your labels to arrays before calling model.fit()
:
train_x = np.asarray(train_x)
train_y = np.asarray(train_y)
validation_x = np.asarray(validation_x)
validation_y = np.asarray(validation_y)
Solution 3
If you encounter this problem while dealing with a custom generator inheriting from the keras.utils.Sequence
class, you might have to make sure that you do not mix a Keras
or a tensorflow - Keras
-import.
This might especially happen when you have to switch to a previous tensorflow
version for compatibility (like with cuDNN
).
If you for example use this with a tensorflow
-version > 2...
from keras.utils import Sequence
class generatorClass(Sequence):
def __init__(self, x_set, y_set, batch_size):
...
def __len__(self):
...
def __getitem__(self, idx):
return ...
... but you actually try to fit this generator in a tensorflow
-version < 2, you have to make sure to import the Sequence
-class from this version like:
keras = tf.compat.v1.keras
Sequence = keras.utils.Sequence
class generatorClass(Sequence):
...
Solution 4
I had a similar problem. In my case it was a problem that I was using a tf.keras.Sequential
model but a keras
generator.
Wrong:
from keras.preprocessing.sequence import TimeseriesGenerator
gen = TimeseriesGenerator(...)
Correct:
gen = tf.keras.preprocessing.sequence.TimeseriesGenerator(...)
Solution 5
This error occured when I updated tensorflow from 1.x to 2.x It was solved after changing my import from
import keras
to
import tensorflow.keras as keras
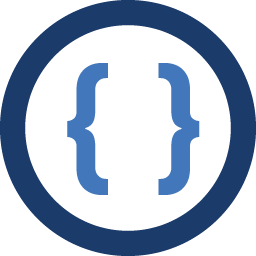
Admin
Updated on July 16, 2022Comments
-
Admin almost 2 years
While running a sentdex tutorial script of a cryptocurrency RNN, link here
YouTube Tutorial: Cryptocurrency-predicting RNN Model,
but have encountered an error when attempting to train the model. My tensorflow version is 2.0.0 and I'm running python 3.6. When attempting to train the model I receive the following error:
File "C:\python36-64\lib\site-packages\tensorflow_core\python\keras\engine\training.py", line 734, in fit use_multiprocessing=use_multiprocessing) File "C:\python36-64\lib\site-packages\tensorflow_core\python\keras\engine\training_v2.py", line 224, in fit distribution_strategy=strategy) File "C:\python36-64\lib\site-packages\tensorflow_core\python\keras\engine\training_v2.py", line 497, in _process_training_inputs adapter_cls = data_adapter.select_data_adapter(x, y) File "C:\python36-64\lib\site-packages\tensorflow_core\python\keras\engine\data_adapter.py", line 628, in select_data_adapter _type_name(x), _type_name(y))) ValueError: Failed to find data adapter that can handle input: <class 'numpy.ndarray'>, (<class 'list'> containing values of types {"<class 'numpy.float64'>"})
Any advice would be greatly appreciated!
-
EliadL almost 4 yearsWhat's the difference here?
-
Alexandre Huat over 3 yearsI don’t see how Keras would accept pandas.DataFrame but not numpy.ndarray
-
John Smith about 3 yearsOne uses plain keras, while the other uses tf.keras.
-
Aelius almost 3 yearsThis was also my case,
keras
was imported both directly through statements likefrom keras import layers
and viaimport tensorflow.keras
. Ensure to stay consistent with the imports addingtensorflow.
before each keras import or removing it.