TextField inside of Row causes layout exception: Unable to calculate size
Solution 1
(I assume you're using a Row
because you want to put other widgets beside the TextField
in the future.)
The Row
widget wants to determine the intrinsic size of its non-flexible children so it knows how much space that it has left for the flexible ones. However, TextField
doesn't have an intrinsic width; it only knows how to size itself to the full width of its parent container. Try wrapping it in a Flexible
or Expanded
to tell the Row
that you're expecting the TextField
to take up the remaining space:
new Row(
children: <Widget>[
new Flexible(
child: new TextField(
decoration: const InputDecoration(helperText: "Enter App ID"),
style: Theme.of(context).textTheme.body1,
),
),
],
),
Solution 2
You get this error because TextField
expands in horizontal direction and so does the Row
, so we need to constrain the width of the TextField
, there are many ways of doing it.
Use
Expanded
Row( children: <Widget>[ Expanded(child: TextField()), OtherWidget(), ], )
Use
Flexible
Row( children: <Widget>[ Flexible(child: TextField()), OtherWidget(), ], )
Wrap it in
Container
orSizedBox
and providewidth
Row( children: <Widget>[ SizedBox(width: 100, child: TextField()), OtherWidget(), ], )
Solution 3
you should use Flexible to use a Textfield inside a row.
new Row(
children: <Widget>[
new Text("hi there"),
new Container(
child:new Flexible(
child: new TextField( ),
),//flexible
),//container
],//widget
),//row
Solution 4
The solution is to wrap your Text()
inside one of the following widgets:
Either Expanded
or Flexible
. So, your code using Expanded will be like:
Expanded(
child: TextField(
decoration: InputDecoration(
hintText: "Demo Text",
hintStyle: TextStyle(fontWeight: FontWeight.w300, color: Colors.red)
),
),
),
Solution 5
As @Asif Shiraz mentioned I had same issue and solved this by Wrapping Column in a Flexible, here like this,,
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new Scaffold(
body: Row(
children: <Widget>[
Flexible(
child: Column(
children: <Widget>[
Container(
child: TextField(),
)
//container
],
))
],
mainAxisAlignment: MainAxisAlignment.spaceBetween,
),
));
}
}
Related videos on Youtube
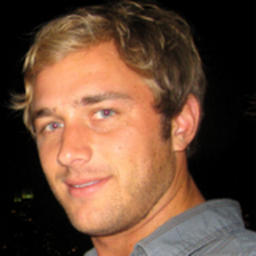
Matthew Smith
Updated on April 25, 2022Comments
-
Matthew Smith about 2 years
I’m getting a rendering exception that I don’t understand how to fix. I’m attempting to create a column that has 3 rows.
Row [Image]
Row [TextField ]
Row [Buttons]
Here is my code to build the container:
Container buildEnterAppContainer(BuildContext context) { var container = new Container( padding: const EdgeInsets.all(8.0), child: new Column( mainAxisAlignment: MainAxisAlignment.start, children: <Widget>[ buildImageRow(context), buildAppEntryRow(context), buildButtonRow(context) ], ), ); return container; }
and my buildAppEntryRow code for the text container
Widget buildAppEntryRow(BuildContext context) { return new Row( children: <Widget>[ new TextField( decoration: const InputDecoration(helperText: "Enter App ID"), style: Theme.of(context).textTheme.body1, ) ], ); }
When I run I get the following exception:
I/flutter ( 7674): BoxConstraints forces an infinite width. I/flutter ( 7674): These invalid constraints were provided to RenderStack's layout() function by the following I/flutter ( 7674): function, which probably computed the invalid constraints in question: I/flutter ( 7674): RenderConstrainedBox.performLayout (package:flutter/src/rendering/proxy_box.dart:256:13) I/flutter ( 7674): The offending constraints were: I/flutter ( 7674): BoxConstraints(w=Infinity, 0.0<=h<=Infinity)
If i change buildAppEntryRow to just a TextField instead like this
Widget buildAppEntryRow2(BuildContext context) { return new TextField( decoration: const InputDecoration(helperText: "Enter App ID"), style: Theme.of(context).textTheme.body1, ); }
I no longer get the exception. What am I missing with the Row implementation that is causing it to not be able to calculate the size of that row?
-
Paresh Mangukiya almost 4 yearsSee this to solve the issue above: stackoverflow.com/a/63698894/10563627
-
-
dragonfly02 over 5 yearsShouldn't this be on flutter doc somewhere?
-
Rap over 5 years@stt106 it is --> flutter.io/docs/development/ui/layout/box-constraints But I agree, it isn't easy to find. Nor do they make the solution as obvious as Collin Jackson did above.
-
Felipe Augusto over 5 yearsI think you don't need the
Container
, you can use theFlexible
directly. -
CircleOnCircles almost 5 yearsThis recommendation is so useful. When you have multiple
TextField
in one row. -
Hasen almost 5 yearsUsing this method breaks
mainAxisAlignment
for the Row widget. With two text widgets there's no problem but with a text widget and a Textfield widget contained inFlexible
it gets aligned to the left with no spacing. -
user3249027 over 4 yearsdoesn't solve the question without adding
width: n
-
Istiaque Ahmed over 4 yearsMay I ask you to take a look at a Flutter related question here : stackoverflow.com/questions/60565658/… ?
-
Magnus over 3 yearsFor some reason this small change required a Flutter Hot Restart (not just Hot Reload) in order to make the layout exception go away in my case.
-
Murat Özbayraktar over 3 yearsIn Row Flexible is perfect. Expanded has fixed height and i crash validation text
-
Johan over 2 yearsevery flutter student must watch this thread or die.