Textfield returning null; bypassing await
Just Add await keyword before showDialog()
and add return statement at last as shown in the below code.
Future<String> changeName() async {
final _controller = TextEditingController();
await showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
shape:
RoundedRectangleBorder(borderRadius: BorderRadius.circular(15)),
title: Text('Change counter name'),
content: TextField(
controller: _controller,
onSubmitted: (str) {
//Navigator.of(context).pop(str);
},
),
actions: <Widget>[
FlatButton(
onPressed: () {
Navigator.of(context).pop(_controller.text.toString());
},
child: Text(
'Confirm',
style: TextStyle(
fontSize: 18.0,
),
),
),
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text(
'Cancel',
style: TextStyle(
fontSize: 18.0,
),
),
),
],
);
},
);
return _controller.text;
}
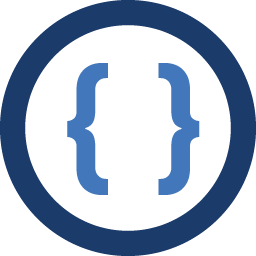
Admin
Updated on December 22, 2022Comments
-
Admin over 1 year
Im making a counter app and want to be able to change the title of the appbar by taking input from the user using an popup input box.
I am trying to have the user input a name(string) in an AlertDialog with textfield in it and trying to recieve the string in the main page by defining a function for the popup which returns the inputted string when confirm button is pressed. I'm assigning a variable to this function using await in the onPressed() of the main page and then displaying the string variable change in a snackbar. however, the snackbar is appearing as soon i tap on the textfield without even submitting or hitting the confirm button. it looks like either the await isnt working or for some reason the Alertdialog's parent function is returning null as soon as I tap on the textfield.
import 'package:flutter/material.dart'; void main() => runApp(MaterialApp( home: CounterApp(), )); class CounterApp extends StatefulWidget { @override _CounterAppState createState() => _CounterAppState(); } class _CounterAppState extends State<CounterApp> { int num = 0; String name = 'MyCounter-1'; Future<String> changeName() async { final _controller = TextEditingController(); showDialog( context: context, builder: (BuildContext context) { return AlertDialog( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(15) ), title: Text('Change counter name'), content: TextField( controller: _controller, onSubmitted: (str) { //Navigator.of(context).pop(str); }, ), actions: <Widget>[ FlatButton( onPressed: () { Navigator.of(context).pop(_controller.text.toString()); }, child: Text( 'Confirm', style: TextStyle( fontSize: 18.0, ), ), ), FlatButton( onPressed: () { Navigator.of(context).pop(); }, child: Text( 'Cancel', style: TextStyle( fontSize: 18.0, ), ), ), ], ); }, ); } void snackBar(BuildContext context, String string) { final snackbar = SnackBar(content: Text('Counter renamed to $string'),); Scaffold.of(context).showSnackBar(snackbar); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(name), centerTitle: true, actions: <Widget>[ IconButton( onPressed: () { setState(() { num = 0; }); }, icon: Icon( Icons.refresh, size: 35.0, ), ), Builder( builder: (context) => IconButton( onPressed: () async { setState(() async { name = await changeName(); snackBar(context, name); }); }, icon: Icon( Icons.edit, size: 35.0, ), ), ), ], ), body: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.stretch, children: <Widget>[ Expanded( flex: 2, child: Container( child: Center(child: Text( '$num', style: TextStyle( fontSize: 75, ), )), ), ), Padding( padding: const EdgeInsets.fromLTRB(20, 0, 20, 10), child: RaisedButton( onPressed: () { setState(() { num += 1; }); }, shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(25.0) ), color: Colors.blue, child: Text( '+', style: TextStyle( fontSize: 100.0, ), ), ), ), Padding( padding: const EdgeInsets.fromLTRB(20, 0, 20, 20), child: RaisedButton( onPressed: () { setState(() { num -= 1; }); }, shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(25.0) ), color: Colors.grey, child: Text( '-', style: TextStyle( fontSize: 100.0, ), ), ), ) ], ), ); } }
As Soon as I tap on the edit button, this happens: Image of problem