The operator '[]' isn't defined for the type 'Iterable<Meal>'. Try defining the operator '[]'.dartundefined_operator
You are passing named arguments to a constructor that takes positional arguments instead.
Change:
import 'package:flutter/material.dart';
import '../models/meal.dart';
class MealItem extends StatelessWidget {
final String title;
final String imageUrl;
final int duration;
final Complexity complexity;
final Affordability affordability;
// use parenthesis '{}' to wrap the constructor arguments to make them named arguments
MealItem({
this.title,
this.imageUrl,
this.duration,
this.complexity,
this.affordability
});
}
}
or simply remove the names and pass positional arguments when creating the MealItem
Also: Positional arguments are required
by default, but named arguments are not. After changing them to named arguments, don't forget to mark them as @required
if you need
Also use toList()
here:
final categoryMeals = DUMMY_MEALS.where((meal) {
return meal.categories.contains(categoryId);
}).toList();
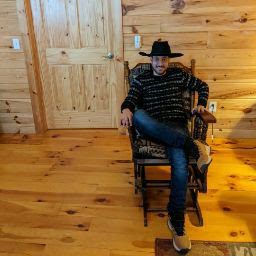
Abdessamad Jadid
Updated on December 22, 2022Comments
-
Abdessamad Jadid over 1 year
im trying return constractor of the class MealItem inside another widget in another class i did the import correctly and i got this
Error : The operator '[]' isn't defined for the type 'Iterable'. Try defining the operator '[]'.dartundefined_operator
Here the MealItem class
import 'package:flutter/material.dart'; import '../models/meal.dart'; class MealItem extends StatelessWidget { final String title; final String imageUrl; final int duration; final Complexity complexity; final Affordability affordability; MealItem( this.title, this.imageUrl, this.duration, this.complexity, this.affordability ); } }
Here is the Error in the CategoryMealsScreen class
import 'package:flutter/material.dart'; import '../widgets/meal_item.dart'; import '../models/dummy_data.dart'; class CategoryMealsScreen extends StatelessWidget { static const routeName = '/CategoriesScreen'; //final String categoryId; //final String categoryTitle; //CategoryMealsScreen(this.categoryId,this.categoryTitle); @override Widget build(BuildContext context) { final routeArgs = ModalRoute.of(context).settings.arguments as Map<String, String>; final categoryTitle = routeArgs['title']; final categoryId = routeArgs['id']; final categoryMeals = DUMMY_MEALS.where((meal) { return meal.categories.contains(categoryId); }); return Scaffold( appBar: AppBar(title: Text(categoryTitle)), body: ListView.builder( itemBuilder: (ctx, index) { return MealItem( title : categoryMeals[index].title, imageUrl: categoryMeals[index].imageUrl, duration: categoryMeals[index].duration, complexity: categoryMeals[index].complexity, affordability: categoryMeals[index].affordability ); }, itemCount: categoryMeals.length, ), ); } }
here is the IDE errors using vsCode :
The operator '[]' isn't defined for the type 'Iterable<Meal>'. Try defining the operator '[]'.
any Help will be appreciated
-
Abdessamad Jadid almost 4 yearsThanks for Help but i still have an error in the index in the CategoryMealsScreen
-
pedro pimont almost 4 yearsso you have more than one error, and the one that's left isn't in the code you shared
-
pedro pimont almost 4 years@AbdessamadJadid it probably has something to do with the categoryMeals, try pasting some of the code where you define it
-
Abdessamad Jadid almost 4 yearsDone, i add the CategoryMealsItem class and the error message below
-
pedro pimont almost 4 years@AbdessamadJadid sorry for the delay, I updated the answer to include the rest of the solution