The type arguments for method cannot be inferred from the usage. Try specifying the type arguments explicitly
Solution 1
This fails to compile because the key and value type of the dictionary needs to be the same, while yours are different. You could change the definition of the method to require a string key type:
public static bool AddItemToCache<T>(string key, Dictionary<string, T> cacheItem, TimeSpan ts)
{
if (!IsCached(key))
{
System.Web.HttpRuntime.Cache.Insert(key, cacheItem, null, Cache.NoAbsoluteExpiration, ts);
return IsCached(key);
}
return true;
}
Solution 2
Change the parameter in your method signature from
Dictionary<T, T> cacheItem
to
Dictionary<TKey, TValue> cacheItem
T, T implies that the key and the value have the same type.
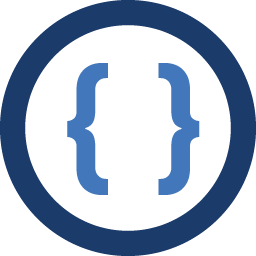
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I've got a linq query that I am returning a
Dictionary<int, string>
from. I've got an overloaded caching method, that I have created that will take aDictionary<T,T>
item as one of the arguments. I've got some other methods in this class that takeList<T>
andT[]
without issue. But this one method, refuses to compile with the error message of the thread subject.This is my code for the caching class:
public static bool AddItemToCache<T>(string key, Dictionary<T, T> cacheItem, DateTime dt) { if (!IsCached(key)) { System.Web.HttpRuntime.Cache.Insert(key, cacheItem, null, dt, TimeSpan.Zero); return IsCached(key); } return true; } public static bool AddItemToCache<T>(string key, Dictionary<T, T> cacheItem, TimeSpan ts) { if (!IsCached(key)) { System.Web.HttpRuntime.Cache.Insert(key, cacheItem, null, Cache.NoAbsoluteExpiration, ts); return IsCached(key); } return true; }
and this is the linq query that is failing to compile:
private Dictionary<int, string> GetCriteriaOptions() { Dictionary<int, string> criteria = new Dictionary<int, string>(); string cacheItem = "NF_OutcomeCriteria"; if (DataCaching.IsCached(cacheItem)) { criteria = (Dictionary<int, string>)DataCaching.GetItemFromCache(cacheItem); } else { Common config = new Common(); int cacheDays = int.Parse(config.GetItemFromConfig("CacheDays")); using (var db = new NemoForceEntities()) { criteria = (from c in db.OutcomeCriterias where c.Visible orderby c.SortOrder ascending select new { c.OutcomeCriteriaID, c.OutcomeCriteriaName }).ToDictionary(c => c.OutcomeCriteriaID, c => c.OutcomeCriteriaName); if ((criteria != null) && (criteria.Any())) { bool isCached = DataCaching.AddItemToCache(cacheItem, criteria, DateTime.Now.AddDays(cacheDays)); if (!isCached) { ApplicationErrorHandler.LogException(new ApplicationException("Unable to cache outcome criteria"), "GetCriteriaOptions()", null, ErrorLevel.NonCritical); } } } } return criteria; }
It's the line isCached = DataCaching..... that I am getting the error. I've tried casting it to a dictionary (
Dictionary<int, string>
), doing a.ToDictionary()
, but nothing works.Anyone got any ideas?