$this->post codeigniter don't work with rest api
12,584
Solution 1
json data you can't get through post method you have to use like this
require(APPPATH . '/libraries/REST_Controller.php');
class user_api extends REST_Controller {
function user_post() {
$params = json_decode(file_get_contents('php://input'), TRUE);
$user = array(
'id' => null,
'firstname' => $params['firstname'],
'lastname' => $params['lastname']
);
$result = $this->user_model->insert($user);
if ($result) {
$this->response($result, 200); // 200 being the HTTP response code
} else {
$this->response(NULL, 404);
}
}
}
Solution 2
//You should use
$array=array(
'firstName' => $this->input->post("firstName"),
'lastName' => $this->input->post("lastName")
);
Solution 3
I think you are not using the correct function to get the values you want the posted data but you are trying to get in $this->post('name')
you should use the CI's method to get the values like
$this->input->post('name');
or
$this->input->get('name');
$user = array(
'id' => null,
'firstname' => $this->input->post('firstname'),
'lastname' => $this->input->post('lastname')
);
You should take a look at Input class
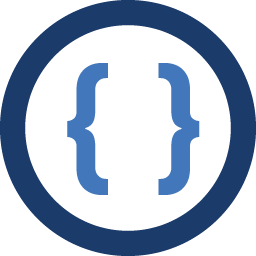
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I tried to work with
$this->post()
to get data sent by post in json format. By I can not get any result of for example$this->post('name')
.This is the code :
<?php require(APPPATH . '/libraries/REST_Controller.php'); class user_api extends REST_Controller { function user_post() { $user = array( 'id' => null, 'firstname' => $this->post('firstname'), 'lastname' => $this->post('lastname') ); $result = $this->user_model->insert($user); if ($result) { $this->response($result, 200); // 200 being the HTTP response code } else { $this->response(NULL, 404); } } } ?>
data sent on json format to the link : http://mydomain.com/myapplication/user_api/user :
{"firstname":"test", "lastname":"test" }
There is no data in the database like it can not get data from
$this->post('firstname')
.Any idea ????