tkinter Treeview widget inserting data
You seem to be interested only in how to insert data the user types within Tkinter.Entry()
widgets into ttk.Treeview()
after a Tkinter.Button()
click.
I designed a simple interface to show you how to resolve this. You can adapt my solution to your problem.
Here is how the application demo looks like:
So I set a counter self.i
to name the items. But you can add a label and an entry for this purpose instead and you insert items names similarly to the the other Tkinter.Entry()
entries.
The insertion method is this one:
def insert_data(self):
"""
Insertion method.
"""
self.treeview.insert('', 'end', text="Item_"+str(self.i),
values=(self.dose_entry.get() + " mg",
self.modified_entry.get()))
# Increment counter
self.i = self.i + 1
May be the main trick here is to retrieve the data the user types using get()
method which thing is represented by self.dose_entry.get()
and self.dose_modified.get()
actions.
This is done, you need now to bind this method to the button to be pressed to trigger the insertion action using the command
option:
self.submit_button = Tkinter.Button(self.parent, text="Insert",
command=self.insert_data)
Full program:
'''
Created on Mar 21, 2016
@author: Bill Begueradj
'''
try:
import Tkinter
import ttk
except ImportError: # Python 3
import tkinter as Tkinter
import tkinter.ttk as ttk
class Begueradj(Tkinter.Frame):
'''
classdocs
'''
def __init__(self, parent):
'''
Constructor
'''
Tkinter.Frame.__init__(self, parent)
self.parent=parent
self.initialize_user_interface()
def initialize_user_interface(self):
"""Draw a user interface allowing the user to type
items and insert them into the treeview
"""
self.parent.title("Canvas Test")
self.parent.grid_rowconfigure(0, weight=1)
self.parent.grid_columnconfigure(0, weight=1)
self.parent.config(background="lavender")
# Define the different GUI widgets
self.dose_label = Tkinter.Label(self.parent, text="Dose:")
self.dose_entry = Tkinter.Entry(self.parent)
self.dose_label.grid(row=0, column=0, sticky=Tkinter.W)
self.dose_entry.grid(row=0, column=1)
self.modified_label = Tkinter.Label(self.parent,
text="Date Modified:")
self.modified_entry = Tkinter.Entry(self.parent)
self.modified_label.grid(row=1, column=0, sticky=Tkinter.W)
self.modified_entry.grid(row=1, column=1)
self.submit_button = Tkinter.Button(self.parent, text="Insert",
command=self.insert_data)
self.submit_button.grid(row=2, column=1, sticky=Tkinter.W)
self.exit_button = Tkinter.Button(self.parent, text="Exit",
command=self.parent.quit)
self.exit_button.grid(row=0, column=3)
# Set the treeview
self.tree = ttk.Treeview(self.parent,
columns=('Dose', 'Modification date'))
self.tree.heading('#0', text='Item')
self.tree.heading('#1', text='Dose')
self.tree.heading('#2', text='Modification Date')
self.tree.column('#1', stretch=Tkinter.YES)
self.tree.column('#2', stretch=Tkinter.YES)
self.tree.column('#0', stretch=Tkinter.YES)
self.tree.grid(row=4, columnspan=4, sticky='nsew')
self.treeview = self.tree
# Initialize the counter
self.i = 0
def insert_data(self):
"""
Insertion method.
"""
self.treeview.insert('', 'end', text="Item_"+str(self.i),
values=(self.dose_entry.get() + " mg",
self.modified_entry.get()))
# Increment counter
self.i = self.i + 1
def main():
root=Tkinter.Tk()
d=Begueradj(root)
root.mainloop()
if __name__=="__main__":
main()
Note:
I coded this in Python 2.7, but it should work if you're using Python 3.x.
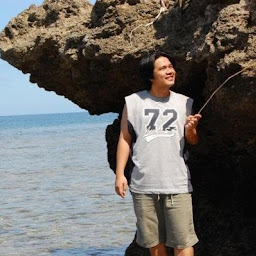
marco alexis
Updated on May 26, 2020Comments
-
marco alexis almost 4 years
This is my sample code. I want the items typed in the entry to be inserted to the treeview when the enter button is pressed. Im new to python and tkinter and there is not much tuts about treeview.
class PurchaseEntry(tk.Frame): def __init__(self, parent, controller): tk.Frame.__init__(self, parent) self.controller = controller PurchaseEntry.configure(self, bg='white') label = ttk.Label(self, text='Purchase Entry', font=LARGE_FONT2) label.grid(row=0, columnspan=3, sticky='w') purchase_entry = ttk.Label(self, text='Purchase Entry:') purchase_entry.grid(row=1, column=0) self.entry_val = tk.StringVar() self.entry_1 = ttk.Entry(self, width=100, textvariable=self.entry_val) self.entry_1.grid(row=1, column=2, columnspan=2, sticky='w') self.entry_1.focus() self.entry_btn = ttk.Button(self,text='Enter', command=self.insert_value) self.entry_btn.grid(row=1, column=4, columnspan=2, sticky='w') self.chat1 = ttk.Treeview(self) chat1 = ttk.Treeview( self, height=28, columns=('dose', 'date modified'), selectmode="extended") chat1.heading('#0', text='item', anchor=tk.CENTER) chat1.heading('#1', text='dose', anchor=tk.CENTER) chat1.heading('#2', text='date modified', anchor=tk.CENTER) chat1.column('#1', stretch=tk.YES, minwidth=50, width=100) chat1.column('#2', stretch=tk.YES, minwidth=50, width=120) chat1.column('#0', stretch=tk.YES, minwidth=50, width=400) chat1.grid(row=2, column=2, columnspan=4, sticky='nsew') def insert_value(self): value = self.entry_val.get() # Inserts data written in the entry box to the treeview widget when Enter button is pressed. # Clears the Entry box, ready for another data entry. self.entry_1.delete(0, 'end') self.chat1.insert('WHAT SHOULD I GIVE AS AN ARGUMENT?')
What should I pass as an argument? Or is treeview the right widget for this or can someone suggest a widget suitable for this issue? thanks