To check whether a number is multiple of second number
12,767
Solution 1
You are using the binary AND operator &
; you want the boolean AND operator here, and
:
x and (y % x) == 0
Next, you want to get your inputs converted to integers:
x = int(input("enter a number :"))
y = int(input("enter its multiple :"))
You'll get a NameError
for that end
expression on a line, drop that altogether, Python doesn't need those.
You can test for just x
; in a boolean context such as an if
statement, a number is considered to be false if 0:
if x and y % x == 0:
Your function is_multiple()
should probably just return a boolean; leave printing to the part of the program doing all the other input/output:
def is_multiple(x, y):
return x and (y % x) == 0
print("A program in python")
x = int(input("enter a number :"))
y = int(input("enter its multiple :"))
if is_multiple(x, y):
print("true")
else:
print("false")
That last part could simplified if using a conditional expression:
print("A program in python")
x = int(input("enter a number :"))
y = int(input("enter its multiple :"))
print("true" if is_multiple(x, y) else "false")
Solution 2
Some things to mention:
- Conditions with
and
, not&
(binary operator) - Convert input to numbers (for example using
int()
) - you might also want to catch if something other than a number is entered
This should work:
def is_multiple(x,y):
if x != 0 and y%x == 0:
print("true")
else:
print("false")
print("A program in python")
x = int(input("enter a number :"))
y = int(input("enter its multiple :"))
is_multiple(x, y)
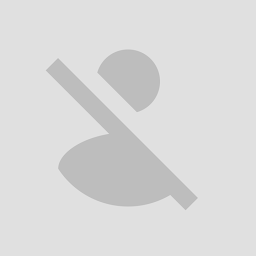
Author by
Vikranth Inti
Updated on June 15, 2022Comments
-
Vikranth Inti almost 2 years
I want to check whether a number is multiple of second. What's wrong with the following code?
def is_multiple(x,y): if x!=0 & (y%x)==0 : print("true") else: print("false") end print("A program in python") x=input("enter a number :") y=input("enter its multiple :") is_multiple(x,y)
error:
TypeError: not all arguments converted during string formatting