to edit a specific line in a textfile using java program
26,091
Just created an example for you
public static void main(String args[]) {
try {
// Open the file that is the first
// command line parameter
FileInputStream fstream = new FileInputStream("d:/new6.txt");
BufferedReader br = new BufferedReader(new InputStreamReader(fstream));
String strLine;
StringBuilder fileContent = new StringBuilder();
//Read File Line By Line
while ((strLine = br.readLine()) != null) {
// Print the content on the console
System.out.println(strLine);
String tokens[] = strLine.split(" ");
if (tokens.length > 0) {
// Here tokens[0] will have value of ID
if (tokens[0].equals("2")) {
tokens[1] = "betty-updated";
tokens[2] = "499";
String newLine = tokens[0] + " " + tokens[1] + " " + tokens[2] + " " + tokens[3];
fileContent.append(newLine);
fileContent.append("\n");
} else {
// update content as it is
fileContent.append(strLine);
fileContent.append("\n");
}
}
}
// Now fileContent will have updated content , which you can override into file
FileWriter fstreamWrite = new FileWriter("d:/new6.txt");
BufferedWriter out = new BufferedWriter(fstreamWrite);
out.write(fileContent.toString());
out.close();
//Close the input stream
in.close();
} catch (Exception e) {//Catch exception if any
System.err.println("Error: " + e.getMessage());
}
}
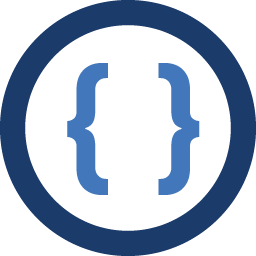
Author by
Admin
Updated on August 09, 2022Comments
-
Admin over 1 year
Ok, say I have a text file called "people.txt", and it contains the following information:
1 adam 20 M 2 betty 49 F 3 charles 9 M 4 david 22 M 5 ethan 41 M 6 faith 23 F 7 greg 22 M 8 heidi 63 F
Basically, the first number is the ID of the person, then comes the person's name, age and gender. Say I want to replace line 2, or the person with ID number 2 with different values. Now, I know I cant use
RandomAccessFile
for this because the names are not always the same number of bytes, neither are the ages. While searching random Java forums, I found thatStringBuilder
orStringBuffer
should suffice for my needs, but I'm not sure how to implement either. Can they be used to directly write to the text file? I want this to work directly from user input. -
Admin almost 12 yearsThanks Attila........... I done creatin file... like insertion.... i dint know how to delete a specific line from that text file by id number...... please help
-
user2032201 almost 12 yearsIf you need to delete the line, then just don't write it out (e.g. don't store it in the list of lines you write out at the end).
-
Admin almost 12 yearsi made the line deletion...... what should i do for updating a specific line....????
-
Admin almost 12 yearsI read some steps in a site... 1. Open the current file for reading. 2. Open a new file for writing. 3. Copy the data from the current to the new file upto the point where you want to modify data. 4. Write the modified data to the new file. 5. Copy the rest of the current file to the new file. 6. Close the files. 7. Delete the current file and rename the new file to the name of the current file. i dont know how to do all these
-
Rahul Agrawal almost 12 years@aleroot has compared ID with startsWith, this logic will fail, when you have multiple ids in file starting with 1, for example 1, 11, 111, 1111, so it will update all these 4 lines.
-
user2032201 almost 12 years@aravindsai2 - check out Rahul's answer. What's left is to have the output at the original location. To do that, move
in.close()
before the creation ofFileWriter
(fstreamWrite
) and change the name of the file opened for writing to the name the original location (change"d:/new6.txt"
to"people.txt"
) -
user2032201 almost 12 yearsYou should move
in.close()
before opening the file for writing, otherwise you might get an exception as the file can be locked