Translate dynamic string using AppLocalizations in flutter
253
You'll need to match you Gender
enum value with your Map
key for your Translations:
enum Gender { male, female }
void main() {
final Map<String, String> translations = {
"male": "مرد",
"female": "زن",
};
Gender.values.map((gender) {
final item = translations
.entries
.firstWhere((element) => element.key == gender.name);
print(item.value);
}).toList();
}
prints out:
مرد
زن
This is the full example scope with my logic:
Following your code, you'll need to find the matching key from the available localizations:
enum Gender { male, female }
final localization = AppLocalizations.of(context)!;
final genders = {
"male": localization.male,
"female": localization.female,
};
Gender.values.map((item) => DropdownMenuItem<Gender>(
value: item,
child: Text(genders.entries
.firstWhere((entry) => entry.key == item.name)
.value))
.toList());
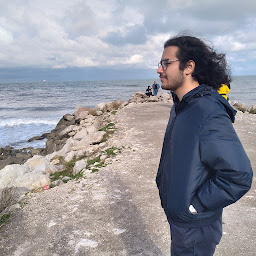
Author by
AmirSina Mashayekh
Computer engineering Bachelor's degree student in Babol Noshirvani University of Technology. Especially interested in embedded systems and microcontrollers (AVR & PIC).
Updated on January 03, 2023Comments
-
AmirSina Mashayekh over 1 year
I want to create a drop down menu for gender.
enum Gender { male, female, }
final localization = AppLocalizations.of(context)!; DropdownButtonFormField( hint: Text(localization.gender), items: Gender.values .map((item) => DropdownMenuItem<Gender>( value: item, // TODO: translate child: Text(item.name), )) .toList(), ),
app_fa.arb:
{ "gender": "جنسیت", "male": "مرد", "female": "زن" }
Everything works well, just I don't know how should I translate 'male' and 'female' and put them in menu items. I need a method which translates a string using keys in
.arb
file.I'm using
flutter_localizations
and main language of my app is Persian. -
AmirSina Mashayekh about 2 yearsthis is good but if I modify the enum, I should modify
genders
too. Isn't there any way to use a string to get translation from arb file? -
Mariano Zorrilla about 2 yearsyou need to match both keys... if not, there's no way for you to map the enum with your localizations. If not, just use DropdownMenuItem<String> and use a map flike the one I created "genders"
-
Mariano Zorrilla about 2 yearslocalization can only give you a value... but the value always change depending the language, that's why you need to create a key for those.
-
Hassan Kanso about 2 yearsit's a workaround, and it will lead to a boilerplate