Translate vector by matrix
Solution 1
It quite easy once you see it.
(New-3d-point) = Metrix-4x4 x (Old-3d-point)
means ...
|x_new| |a1 a2 a3 a4| |x_old|
|y_new| = |b1 b2 b3 b4| x |y_old|
|z_new| |c1 c2 c3 c4| |z_old|
| 1 | |d1 d2 d3 d4| | 1 |
means ...
x_new = a1*x_old + a2*y_old + a3*z_old + a4
y_new = b1*x_old + b2*y_old + b3*z_old + b4
z_new = c1*x_old + c2*y_old + c3*z_old + c4
d1-d4 are there to make '1' so you don't need to use it.
Hope this helps.
Solution 2
You need the maths, though. I will try to be gentle, but if you don't know how to do matrix multiplication, you need to look it up, right now!
First, you need to make your 3D vector into a 4-vector, by adding an extra 1 to it. Computer graphics usually calls this a "w" coordinate (to go with the x, y, and z coordinates).
3D vector: (X,Y,Z) -> 4D vector: (x=X, y=Y, z=Z, w=1)
Then, you can multiply the 4-vector by the 4x4 matrix. What this does depends on the matrix -- but you can make a matrix that translates the vector:
[x,y,z,1] * [1 0 0 0] = [x+a,y+b,z+c,1]
[0 1 0 0]
[0 0 1 0]
[a b c 1]
Unsurprisingly, this kind of matrix is called a translation matrix.
The advantage of doing things this way is that you can also make a rotation matrix, or a scaling matrix, then you can multiply as many of them as you want together into one matrix:
v * M1 * M2 * M3 = v * (M1*M2*M3)
Multiplying a vector by the resulting matrix is equivalent to multiplying by all the component matrices in sequence, which as you might imagine can save you a lot of time and hassle.
Code for multiplying a 4-vector by a 4x4 matrix might be something like:
for(int i=0; i<4; ++i) {
double accumulator= 0.0;
for(int j=0; j<4; ++j) {
accumulator+= rowVectorIn[j]*matrix[j][i]; // matrix is stored by rows
}
rowVectorOut[i]= accumulator;
}
Solution 3
If you treat your (generally 3d) vector (x, y, z) as a four vector (x, y, z, 1) you can do this: w = Av
T
, where T
is the transpose operation (twist a horzontal vector vertical or vice versa) and A
is a correctly chosen matrix, and w
is the translated matrix.
You do have to know how to do matrix multiplication.
To get a transposition by (a, b, c)
and nothing else define A
as:
1 0 0 a
0 1 0 b
0 0 1 c
0 0 0 1
Solution 4
I'm not sure exactly what form your data is coming in but just on first glance it looks like you might be dealing with quaternions.
Without getting too deep into the math, there are a couple ways to represent a three dimensional matrix transform. There are Euler angles and quaternions. Euler angles are definitely simpler but quaternions have the advantage of not being susceptible to gimble lock.
Quaternions of real values in three dimensions are represented with 4x4 matrices which is why I thought this may apply to you. Of course, the authors of the other solutions may be right and what you're dealing with could be something totally different but it might be worth learning about anyway.
Hope this helps. Good luck!
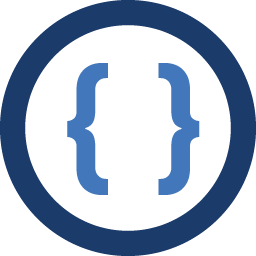
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have a 4*4 matrix and a 3d vector. I need to translate my vector by the matrix.
Not too much crazy maths notation please because I don't understand it.
An example in something close to java would be fab!