Trigger change() event when setting <select>'s value with val() function
Solution 1
I had a very similar issue and I'm not quite sure what you're having a problem with, as your suggested code worked great for me. It immediately (a requirement of yours) triggers the following change code.
$('#selectField').change(function(){
if($('#selectField').val() == 'N'){
$('#secondaryInput').hide();
} else {
$('#secondaryInput').show();
}
});
Then I take the value from the database (this is used on a form for both new input and editing existing records), set it as the selected value, and add the piece I was missing to trigger the above code, ".change()".
$('#selectField').val(valueFromDatabase).change();
So that if the existing value from the database is 'N', it immediately hides the secondary input field in my form.
Solution 2
One thing that I found out (the hard way), is that you should have
$('#selectField').change(function(){
// some content ...
});
defined BEFORE you are using
$('#selectField').val(10).trigger('change');
or
$('#selectField').val(10).change();
Solution 3
The straight answer is already in a duplicate question: Why does the jquery change event not trigger when I set the value of a select using val()?
As you probably know setting the value of the select doesn't trigger the change() event, if you're looking for an event that is fired when an element's value has been changed through JS there isn't one.
If you really want to do this I guess the only way is to write a function that checks the DOM on an interval and tracks changed values, but definitely don't do this unless you must (not sure why you ever would need to)
Added this solution:
Another possible solution would be to create your own .val()
wrapper function and have it trigger a custom event after setting the value through .val()
, then when you use your .val() wrapper to set the value of a <select>
it will trigger your custom event which you can trap and handle.
Be sure to return this
, so it is chainable in jQuery fashion
Solution 4
As jQuery won't trigger native change event but only triggers its own change event. If you bind event without jQuery and then use jQuery to trigger it the callbacks you bound won't run !
The solution is then like below (100% working) :
var sortBySelect = document.querySelector("select.your-class");
sortBySelect.value = "new value";
sortBySelect.dispatchEvent(new Event("change"));
Solution 5
I separate it, and then use an identity comparison to dictate what is does next.
$("#selectField").change(function(){
if(this.value === 'textValue1'){ $(".contentClass1").fadeIn(); }
if(this.value === 'textValue2'){ $(".contentclass2").fadeIn(); }
});
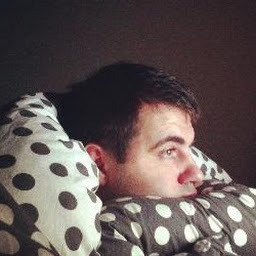
Goran Radulovic
Web Developer, Entrepreneur, co-founder of Active Collab, one of the most popular project management & team collaboration platforms on the market activecollab.com
Updated on January 11, 2020Comments
-
Goran Radulovic over 4 years
What is the easiest and best way to trigger change event when setting the value of select element.
I've expected that executing the following code
$('select#some').val(10);
or
$('select#some').attr('value', 10);
would result in triggering the change event, which i think is pretty logic thing to be done. Right?
Well, it isn't the case. You need to triger change() event by doing this
$('select#some').val(10).change();
or
$('select#some').val(10).trigger('change');
but i'm looking for some solution which would trigger change event as soon as select's value is changed by some javascript code.
-
Con Antonakos over 9 yearsThis is exactly what I needed. What is the best way to discover how to dynamically trigger DOM event handlers? Does jQuery document this well? Thank you!
-
David L over 9 yearsI've read several forums on this question, and your answer was the last completion I was looking for. The idea of tracking only works as a mental experiment, obviously it would utterly impractical, but it's illustrative; and the idea of the wrapper is awesome, and thank you for pointing out the "return this" part. Regards.
-
Temitayo almost 9 yearsOld post but this solved my problem, saved me from a long winded brain cracking... You must define the listener before using it. Even in pure JavaScript.
-
Dima R. almost 9 yearsCan you elaborate a little? Isn't the "change()" is there for this reason ?
-
Temitayo almost 9 yearsWell I will say maybe listeners of these sort are not hoisted on load, In my project, I called the
change()
before defining the listener and it didn't work until I moved thechange()
call under the listener and it worked. Well I will say this shouldn't be a problem, it is just a matter of the way you structure your code. -
Jamie M. over 8 yearsThis is what I needed. Took me forever to solve this issue, thanks.
-
Satanand Tiwari about 7 years$("#discount_type") .val(2) .trigger('change');
-
Istiaque Ahmed about 7 yearsWhy won't
$('select').val(value).change()
work all the time ? Any clear explanation ? -
Kamlesh over 4 years$('#add_itp_spt_parent_id').val(add_fpt_val).trigger('change'); $('#add_itp_spt_parent_id').change(); both solutions are not working.
-
Kamlesh over 4 yearsdefined change function BEFORE you are using, solved my problem. Thanks.
-
Kamlesh over 4 yearsBravo!!! "defined change function BEFORE you are using", solved my problem. Thanks dear.