Trim the video to 5 minutes
3,205
Solution 1
You can use following command code to split a video.
final FlutterFFmpeg _flutterFFmpeg = new FlutterFFmpeg();
_flutterFFmpeg
.execute(
'-i videoplayback.mp4 -ss 00:00:50 -t 00:01:30 -c copy smallfile1.mp4')
.then((value) {
print('Got value ');
}).catchError((error) {
print('Error');
});
Start time is -ss 00:00:50
end time is 00:01:30
Solution 2
You can use any ffmpeg
command in execute()
method of flutter-ffmpeg package, to trim first 5 minutes of video
import 'package:flutter_ffmpeg/flutter_ffmpeg.dart';
....
final FlutterFFmpeg _flutterFFmpeg = new FlutterFFmpeg();
_flutterFFmpeg.execute("-ss 00:00:00 -i input.mp4 -to 00:05:00 -c copy output.mp4").then((rc) => print("FFmpeg process exited with rc $rc"));
Solution 3
For getting video information you may can try following code.
fFmpeg.execute('-i video.mp4 -f null /dev/null').then((value) {
print('Got value ');
}).catchError((error) {
print('Error');
});
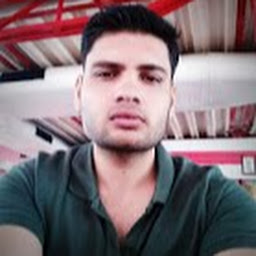
Author by
Sudhansu Joshi
Updated on December 09, 2022Comments
-
Sudhansu Joshi over 1 year
I am working on a demo project on which I have to get only 5 minutes video is there any way to trim the video to 5 minutes only. Currently, I am using FFmpeg flutter to get the video file and its metadata.
-
Sudhansu Joshi about 5 yearsFFmpeg is taking too long for getting metadata of video like 25seconds for 6 minutes video is there any way to cut short this time.
-
Sudhansu Joshi about 5 yearsFFmpeg is taking too long for getting metadata of video like 25seconds for 6 minutes video is there any way to cut short this time
-
Sudhansu Joshi about 5 yearsI have got a path to the video. I want to fetch metadata with the absolute path of the video.
-
Sudhansu Joshi about 5 yearsOnly video name is given in ffmpeg arguments in your code.
-
Santosh Anand about 5 yearsWhere is your file?
-
Parth Bhanderi over 4 yearswhen i run my flutter project i got the error from my pod file ``` [!] Invalid
Podfile
file: no implicit conversion of Hash into String. # from /Volumes/Data/backup/We_Begins/We_Begins_With_Me/ios/Podfile:69 # ------------------------------------------- # plugin_pods.each do |name, path| > symlink = File.join('.symlinks', 'plugins', name) # File.symlink(path, symlink) # ------------------------------------------- ``` -
user969068 over 4 years@ParthBhanderi it is related to
CocoaPods
, could you show complete error log -
Parth Bhanderi over 4 yearsthanks for reply i post my error log in answer bloc.
-
Parth Bhanderi over 4 yearscan you give me example how to use this plugin and what is the input.mp4 and output.mp4
-
b.john almost 4 yearsWhen i trim with ffmpeg, the first few seconds, i get only the sound no video. After few seconds is when the video comes. What could be the issue.