Trying to apply CSS transform in javascript
16,783
You can do it like below. Don't forget to set top/left
to always have the same behavior since translate will apply translation from the position of the element.
var cursor = document.getElementById('cursor');
document.body.addEventListener("mousemove", function(e) {
//storing cursor position as variables
var curX = e.clientX;
var curY = e.clientY;
// I need the code below to be replaced with transform-translate instead of top/left
// I can not get this to work with any other method than top/left
//cursor.style.left = curX - 7 + 'px';
//cursor.style.top = curY - 7 + 'px';
cursor.style.transform = "translate(" + (curX - 7) + "px," + (curY - 7) + "px)";
});
body {
background: orange;
height: 500px;
width: 500px;
}
#cursor {
position: fixed;
top:0;
left:0;
z-index: 20000;
height: 14px;
width: 14px;
background-color: #222;
border-radius: 50%;
pointer-events: none!important;
}
<body>
<div id="cursor"></div>
</body>
You can consider percentage and calc()
if you want this to be dynamic and work with any width/height:
var cursor = document.getElementById('cursor');
document.body.addEventListener("mousemove", function(e) {
//storing cursor position as variables
var curX = e.clientX;
var curY = e.clientY;
// I need the code below to be replaced with transform-translate instead of top/left
// I can not get this to work with any other method than top/left
//cursor.style.left = curX - 7 + 'px';
//cursor.style.top = curY - 7 + 'px';
cursor.style.transform = "translate(calc(" + curX + "px - 50%),calc(" + curY + "px - 50%))";
});
body {
background: orange;
height: 500px;
width: 500px;
margin: 0;
}
#cursor {
position: fixed;
top: 0;
left: 0;
z-index: 20000;
height: 14px;
width: 14px;
background-color: #222;
border-radius: 50%;
pointer-events: none!important;
}
<body>
<div id="cursor"></div>
</body>
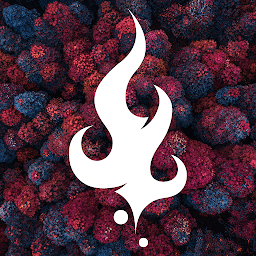
Comments
-
Shape almost 2 years
On my page I have an element that follows users cursor. What I'm trying to do is to use
transform
insteadtop
/left
with plain javascript. Without any libraries or depencies... The problem is that I need to apply values stored in variables to the transform property. I didn't find anything that could help me... Here is my code:var cursor = document.getElementById('cursor'); document.body.addEventListener("mousemove", function(e) { //storing cursor position as variables var curX = e.clientX; var curY = e.clientY; // I need the code below to be replaced with transform-translate instead of top/left // I can not get this to work with any other method than top/left cursor.style.left = curX - 7 + 'px'; cursor.style.top = curY - 7 + 'px'; });
body { background: orange; height: 500px; width: 500px; } #cursor { position: fixed; z-index: 20000; height: 14px; width: 14px; background-color: #222; border-radius: 50%; pointer-events: none!important; }
<body> <div id="cursor"></div> </body>
It's a simple or even a silly question but I have not found anything like this on stackoverflow or google...
-
connexo over 5 yearsIE10, IE11, and Edge < 14 don't support using calc() inside a transform. caniuse.com/#search=calc -> Known Issues