Trying to construct identity matrix?
Solution 1
If numpy is not allowed ... Know this How to define two-dimensional array in python
and do this
def identity(n):
m=[[0 for x in range(n)] for y in range(n)]
for i in range(0,n):
m[i][i] = 1
return m
Solution 2
This is because the way you are initializing matrix
. Each sublist of [[0]*n]*n
is the same list [0]*n
, or in other words, each row of your matrix is a reference to the same underlying row. You can verify this using id
:
> x = [[0]*3]*3
> x
[[0, 0, 0], [0, 0, 0], [0, 0, 0]]
> id(x[0])
140017690403112
> id(x[1])
140017690403112
> id(x[2])
140017690403112
TTherefore, when you assign a value to the i
th row of your matrix, you're assigning it to all rows. So avoid nested list creation using [0]*n
. Instead, use
matrix = [[0]*n for _ in range(n)]
Even simpler, avoid all of this with:
import numpy as np
np.eye(n)
Solution 3
Numpy has this built in, you can just use np.eye(n):
In [1]: import numpy as np
In [2]: x = np.eye(4)
In [3]: x
Out[3]:
array([[ 1., 0., 0., 0.],
[ 0., 1., 0., 0.],
[ 0., 0., 1., 0.],
[ 0., 0., 0., 1.]])
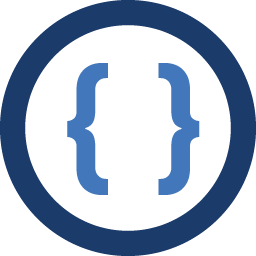
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
Write a function identity(n) that returns the n identity matrix.
For example: identity(3) outputs [[1,0,0][0,1,0][0,0,1]]
I have tried as follow:def identity(n): matrix=[[0]*n]*n i=0 while i<n: matrix[i][i]=1 i+=1 return matrix
Also I tried with range but it did'n work like this
def identity(n): matrix=[[0]*n]*n k=matrix[:] i=0 for i in range(1,n): matrix[i][i]=1 i+=1 return k print(identity(5))
But it output for
n = 5
:[[1, 1, 1, 1, 1], [1, 1, 1, 1, 1], [1, 1, 1, 1, 1], [1, 1, 1, 1, 1], [1, 1, 1, 1, 1]]