Trying to MPI_Send and MPI_Recv char array getting garbage
14,841
You are passing a char**
to MPI_Send
instead of a char*
this causes memory corruption, or in your case the garbled output you are getting. Everything should be fine if you use
MPI_Send (temp, length+1, MPI_CHAR, 0, 1, MPI_COMM_WORLD);
(note the removed &
in front of the first argument, temp
.)
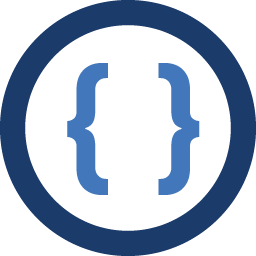
Author by
Admin
Updated on July 25, 2022Comments
-
Admin almost 2 years
I'm trying to create a log file from each processor and then send that to the root as a char array. I first send the length and then I send the data. The length sends fine, but the data is always garbage! Here is my code:
MPI_Barrier (MPI_COMM_WORLD); string out = ""; MPI_Status status[2]; MPI_Request reqs[num_procs]; string log = "TEST"; int length = log.length(); char* temp = (char *) malloc(length+1); strcpy(temp, log.c_str()); if (my_id != 0) { MPI_Send (&length, 1, MPI_INT, 0, 1, MPI_COMM_WORLD); MPI_Send (&temp, length+1, MPI_CHAR, 0, 1, MPI_COMM_WORLD); } else { int length; for (int i = 1; i < num_procs; i++) { MPI_Recv (&length, 2, MPI_INT, i, 1, MPI_COMM_WORLD, &status[0]); char* rec_buf; rec_buf = (char *) malloc(length+1); MPI_Recv (rec_buf, length+1, MPI_CHAR, i, 1, MPI_COMM_WORLD, &status[1]); out += rec_buf; free(rec_buf); } } MPI_Barrier (MPI_COMM_WORLD); free(temp);