Trying to use DropDownListFor without model getting "tree may not contain dynamic operation" error
I suggest some changes in your solution:
Instead of
DropDownListFor()
use justDropDownList()
@Html.DropDownList("CultureCode", new SelectList(db.CultureCodes, "ID", "DisplayName"))
Instead of accessing your database data in your view... which is very out of the standard and you are coupling the Views (usually HTML) with the database... you should put the query in your controller and put the data in the ViewBag collection.
So, in your Layout, instead of the code I suggested above, you should use:
@Html.DropDownList("CultureCode", (SelectList)ViewBag.Codes, "Select one...")
In your controller you load it as follow:
ViewBag.Codes = new SelectList(db.CultureCodes, "ID", "DisplayName");
EDIT:
You can do an action filter, to load or inject the CultureCodes
in the ViewBag
:
public class IncludeCultureCodesAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
var controller = filterContext.Controller;
// IController is not necessarily a Controller
if (controller != null)
{
var db = new YourContext();
controller.ViewBag.Codes = new SelectList(db.CultureCodes, "ID", "DisplayName"));;
}
}
}
Then, in your Controller actions... you can decorate them with [IncludeCultureCodes]
. So, the action with that attribute will load the collection of codes.
But I think is a better approach to load one time the layout (on Home/Index for example) and then use partial views. This way you only reload the layout going back to home... or other full view calls.
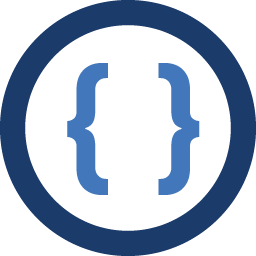
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I am trying to use a
DropDownListFor<>
in my LayoutTemple so I don't have access to a model. So what I did was in the@{}
block at the top of the page I addedFFInfo.DAL.SoloClassesContext db = new FFInfo.DAL.SoloClassesContext();
which calls an instance of the DBContext with the class I want to use. I then placed the List where I wanted using@Html.DropDownListFor( m => m.ID, new SelectList(db.CultureCodes, "ID", "DisplayName"));
but when I run the code I get an error for the line
m => m.ID
. The error given is:An expression tree may not contain a dynamic operation
I have never used this type of dropdown and am very new to MVC. Can anyone tell me what I am doing wrong and how to fix it?