Tutorial first time you enter into an app?
Solution 1
I encountered this thread while looking for a solution for running a tutorial only at the first time (as rbaleksandar suggested), so in case it will be helpful for someone someday, here's a template of a solution that works for me (using the SharedPreferences
API):
@Override
protected void onResume() {
super.onResume();
String tutorialKey = "SOME_KEY";
Boolean firstTime = getPreferences(MODE_PRIVATE).getBoolean(tutorialKey, true);
if (firstTime) {
runTutorial(); // here you do what you want to do - an activity tutorial in my case
getPreferences(MODE_PRIVATE).edit().putBoolean(tutorialKey, false).apply();
}
}
EDIT - BONUS - If you're into app tutorial - I'm messing now with the ShowcaseView library (which is amazing - try it out). Apparently they have some shortcut for that issue using a method called singleShot(long)
- its input is a key for the SharedPreferences
, and it does the exact same thing - runs only in the first activation. Example of usage (taken from here):
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_single_shot);
Target viewTarget = new ViewTarget(R.id.button, this);
new ShowcaseView.Builder(this)
.setTarget(viewTarget)
.setContentTitle(R.string.title_single_shot)
.setContentText(R.string.R_string_desc_single_shot)
.singleShot(42)
.build();
}
Solution 2
You could always code your own solution, but, let us not reinvent the wheel.
Check this Android Library:
It allows you to add pointers in your screen, so the user knows where is he supposed to touch next.
It's pretty easy to use, you only need to point to the element you want the user to touch.
From the doc:
Let's say you have a button like this where you want user to click on:
Button button = (Button)findViewById(R.id.button);
You can add the tutorial pointer on top of it by:
TourGuide mTourGuideHandler = TourGuide.init(this).with(TourGuide.Technique.Click)
.setPointer(new Pointer())
.setToolTip(new ToolTip().setTitle("Welcome!").setDescription("Click on Get Started to begin..."))
.setOverlay(new Overlay())
.playOn(button);
Hope this helps!
Solution 3
As far as I understand the question is not How do I create a tutorial? (as the people who have already posted an answer have concluded) but instead How to show a tutorial upon first launch only?. So here are my two cents on this topic:
I'm not familiar with how your Android app stores its configuration data but I will assume that it's either in a database (SQLite) or a text file (plaintext, YAML, XML - whatever). Add a configuration entry to wherever the app's settings are being stored - something like tutorial_on : false
, tutorial_on : 1
etc. depending on the format the configuration is represented in.
The way configurations work is that whenever an app (or software in general) is launched it has to be loaded in the app itself. So add the following to your app (where and how is up to you and your app design):
- Check
tutorial_on
entry -
If
tutorial_on
is set totrue
/1
whatever2.1 Display tutorial
2.2 Change
tutorial_on
tofalse
/0
whatever2.3 Store the result in your configuration
- Continue using the app
By doing so the first time your app launches the flag responsible for displaying the tutorial will be toggled and afterwards every time you start the app the toggle flag will be read leading to omitting the tutorial.
Personally I would suggest that you an option similar to Don't show tutorial anymore
along with a description how to re-enable it (by triggering some action in that app's menu etc.). This has two major benefits:
- Improved user experience - users like to have control (especially over trivial matters such as showing or hiding a tutorial). Whenever you take the control away from them, they get pissed off.
-
Enable your user to re-learn forgotten things - a general rule of thumb is to create apps that should not burden the user with a lot of stuff to remember. That is why things should be self-explanatory. However sometimes you may want to do that nonetheless. By adding the possibility that the user re-launches (by simply resetting the
tutorial_on
flag and repeating the steps from above) the tutorial allows just that - refreshing a user's memory.
Solution 4
Some links to libraries for creating introduction and/or tutorial screens.
Horizontal cards like Google Now: https://github.com/PaoloRotolo/AppIntro
Tutorial screen: https://github.com/amlcurran/ShowcaseView
Related videos on Youtube
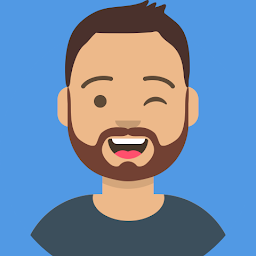
Giovanni Giampaolo
Updated on June 04, 2022Comments
-
Giovanni Giampaolo about 2 years
I'm programming an app using android studio. I want to know in which way I can do a tutorial that users will see only the first time that use the app. Tutorial like image or screenshoots
Can someone help me? Thanks
-
Giovanni Giampaolo over 8 yearsThanks...I like so much the style!
-
waseefakhtar about 6 yearsThose are brilliant solutions! Thanks so much!
-
tekin beyaz over 5 yearsI am having trouble to use this TourGuide on a BottomNavigationView. I guess .playOn does not work on menu items. And compiler gave an error about (TourGuide.Technique.Click) statement. I needed to change it with .CLICK
-
Yousef Gamal about 5 years
setOverlay()
returns void, soplayOn
can't be called after it, however, callingplayOn()
first thensetOverlay()
should work