Twitter Bootstrap 3 - Panels of Equal Height in a Fluid Row
Solution 1
This can be done with CSS flexbox. Only minimal CSS is needed..
.equal {
display: -webkit-flex;
display: flex;
}
Just add .equal
to your .row
and flexbox does the rest.
http://www.codeply.com/go/BZA25rTY45
UPDATE: Bootstrap 4 uses flexbox so there is no need for the additional CSS. http://www.codeply.com/go/0Aq0p6IcHs
Solution 2
Bootstrap's solution - add this css and add the class to your row:
.row-eq-height {
display: -webkit-box;
display: -webkit-flex;
display: -ms-flexbox;
display: flex;
}
It has a couple caveats, as posted at http://getbootstrap.com.vn/examples/equal-height-columns/, but it sounds like it'll be good enough for your case.
I also saw this answer here.
Update for dynamic number of columns
Bootstrap automatically wraps columns into new rows when you add more than can fit in one row, but this flexbox approach breaks this. To get flexbox to wrap, I've found you can do something like this:
-webkit-flex-flow: row wrap;
flex-flow: row wrap;
-webkit-align-content: flex-end;
align-content: flex-end;
This is awesome when you have a dynamic number of columns, or columns that change width according to the screen size. So you can have something like this:
<div class="row row-eq-height">
<div class="col-sm-6 col-md-4">Content...</div>
<div class="col-sm-6 col-md-4">Content...</div>
<div class="col-sm-6 col-md-4">Content...</div>
<div class="col-sm-6 col-md-4">Content...</div>
<div class="col-sm-6 col-md-4">Content...</div>
</div>
And it all lines up the way it's expected. Just watch out - it only works in newer browsers. More info here
Solution 3
If you're going to use the bootstrap grid, I don't think you're going to find an easy way to accomplish this without hacks like the following css.
This basically says. When the screen width is greater than the md
breakpoint in bootstrap, give all the elements with panel-body
class which are direct descendants of the column elements a minimum height of 420px
which happens to be a "magic number" that works with your existing content.
Again, I think this is a really gross solution, but it "works" in a pinch.
@media (min-width: 992px) {
.col-md-4 > .panel > .panel-body {
min-height: 420px;
}
}
Here's a CSS-Tricks article Fluid Width Equal Height Columns which covers various ways (display: table
& flexbox) to accomplish this. However, you might need to step away from the responsive bootstrap grid for this particular task.
Also, here's the Complete Guide to Flexbox
Solution 4
Haven't found any of these CSS methods to work in my case I am using images in my panels too instead I used a simple JQuery function to get the job done
window.onload = function resizePanel(){
var h = $("#panel-2").height();
$("#panel-1").height(h); // add a line like this for each panel you want to resize
}
Where the ids "panel-1" and and "panel-2" are in the panel tag choose the largest panel as the one you use to set h and call the function at the end of you html.
<body onresize = "resizePanel()">
I also make it so the function is called when if the window is resized by adding the onresize attribute to the body
Solution 5
I add top and bottom paddings in style for the lower <div>
.
<div class="xxx" style="padding: 8px 0px 8px 0px">
...
</div>
Because after all each case is different and you have to adjust according to the situation.
Chrome dev tool can be very useful in situations like this.
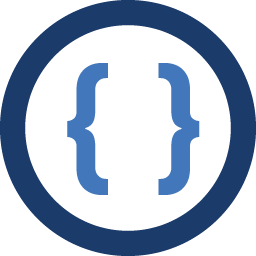
Admin
Updated on December 15, 2020Comments
-
Admin over 3 years
I am new to Bootstrap 3 and I would like to have 3 panels on my landing page of equal height, even though the middle panel has less content. When resized they become the same height, but are not upon initial visit to the page.
I already tried hiding the overflow with CSS and it cuts off the bottom of the panel which isn't what I want, so I'm thinking I need to use jQuery.
Here is my code:
<div class="row-fluid"> <!--begin panel 1 --> <div class="col-md-4"> <div style="text-align:center" class="panel panel-primary"> <div class="panel-heading"> <h1 class="panel-title text-center">Web y Metrícas</h1> </div> <!-- end panel-heading --> <div class="panel-body"> <p> <img style="margin: 0 auto;" class="img-responsive" src="web.png" height="30%" width="30%" alt="Web y Metrícas" /> </p> <p class="text-left lead2">Apoyamos estratégicamente la presencia de tu empresa en el mundo digital, a través de la construcción de recursos web atractivos para tus clientes.</p> <ul class="text-left"> <li>Web Corporativas</li> <li>Tiendas Virtuales</li> <li>Plataformas e-Learning</li> <li>Arquitectura de Información</li> <li>Google Analytics, SEO–SEM</li> <li>Análisis de Competencia Digital</li> <li>Data Mining</li> </ul> <a class="btn btn-primary" href="#">Ver más »</a> </div> <!-- end panel-body --> </div> <!-- end panel-primary --> </div> <!--end col-md-4 --> <!-- begin panel 2 --> <div class="col-md-4"> <div style="text-align:center" class="panel panel-primary"> <div class="panel-heading"> <h1 class="panel-title">Gestíon de Redes Socials</h1> </div> <!-- end panel-heading --> <div class="panel-body"> <p> <img style="margin: 0 auto;" class="img-responsive" src="redes.png" height="30%" width="30%" alt="Gestíon de Redes Socials" /> </p> <p class="text-left lead2">Crear una experiencia de marca excepcional a través de redes es más inteligente, rápida y las comunicaciones sociales serán más eficientes.</p> <ul class="text-left"> <li>Compromiso</li> <li>Publicación</li> <li>Monitoreo</li> <li>Analítica</li> <li>Colaboración</li> <li>CRM</li> <li>Movil</li> </ul> <a class="btn btn-primary" href="#">Ver más »</a> </div> <!-- end panel-body --> </div> <!-- end panel-primary --> </div> <!-- end col-md-4 --> <!--begin panel 3 --> <div class="col-md-4"> <div style="text-align:center" class="panel panel-primary"> <div class="panel-heading"> <h1 class="panel-title">Plan de Medios</h1> </div> <!-- end panel-heading --> <div class="panel-body"> <p> <img style="margin: 0 auto;" class="img-responsive" src="medios.png" height="30%" width="30%" alt="Plan de Medios" /> </p> <p class="text-left lead2">Trabajamos en conjunto con la empresa para reforzar las fortalezas de su organización y las comunicamos de forma integral y con un mensaje claro.</p> <ul class="text-left"> <li>Asesoría Comunicacional</li> <li>RR.PP</li> <li>Presencia de Marca</li> <li>Clipping Digital</li> <li>Manejo de Crisis</li> <li>Lobby</li> <li>Media Training</li> </ul> <a class="btn btn-primary" href="#">Ver más »</a> </div> <!-- end panel-body --> </div> <!-- end panel-primary --> </div> <!-- end col-md-4 --> </div> <!-- end row -->
-
cdonner over 10 yearsI have the same issue and did not find a better way to do this.
-
pascalvgemert about 10 yearsDoesn't work correctly when using image 100% in
.col
-
Alix Axel almost 10 years@Skelly: In your example, if you resize the browser to a lower width, the width of each individual panel changes as well and they become inconsistent (one wider than the other). Is there a way to maintain the width proportional / consistent?
-
Stuart Clark over 9 yearsYou could add to this function so that it automatically find the biggest panel but in my case it wasn't worth the time
-
m4r73n over 9 yearsIt does not work when you also have a
.panel-footer
. -
m79lkm over 8 yearsI put this inside a
@media(min-width:992px)
block and it works beautifully. Thanks! -
LZH almost 8 yearseven though it is not a nice solution, but it works.
-
Grid Trekkor over 7 yearsThis works for equal height, but causes the width of panels to be erratic and/or not 100% of the column.
-
Zim over 7 years@Grid Trekkor The Q is about equal height, and that's what this answer addresses. I'm not seeing the width of panels vary. They remain 100% of the column even when resized. What browser are you using?
-
JB06 over 7 years@GridTrekkor I have the same issue with the width, just add
.panel { width: 100% }
and that resolves it. +1 for the answer, helped me out