Twitter Bootstrap expanding text using the "Collapse" plugin
Solution 1
I got this to work by adding the following JavaScript:
$("div").on("hide", function() {
$(this).prev("div").find("i").attr("class","icon-plus-sign");
$(this).css("display","");
$(this).css("height","5px");
});
$("div").on("show", function() {
$(this).prev("div").find("i").attr("class","icon-minus-sign");
$(this).css("display","inline");
$(this).css("height","5px");
});
And the following CSS:
div[data-toggle="collapse"] {
float: left;
}
Sidenote
I personally would not use the Bootstrap collapse plugin for this. I would use a dedicated plugin like JQuery Expander in combination with Bootstrap icons.
A different example fiddle is here.
Solution 2
I agree with the other post that the collapse plugin is not meant for this. It is actually quite easy to write something that handles this nicely. For example, by wrapping the hidden text in <span class="truncated">
:
<p>
This is the visible part <span class="truncated">this is the hidden part.</span>
</p>
Then hide it, append the hide/show icon, and toggle state:
$('.truncated').hide() // Hide the text initially
.after('<i class="icon-plus-sign"></i>') // Create toggle button
.next().on('click', function(){ // Attach behavior
$(this).toggleClass('icon-minus-sign') // Swap the icon
.prev().toggle(); // Hide/show the text
});
This takes advantage of the priority of the icon-minus-sign
class over the icon-plus-sign
one, but you can add in a toggle for both if it makes you feel safer.
Demo: http://jsfiddle.net/VNdmZ/4/
Related videos on Youtube
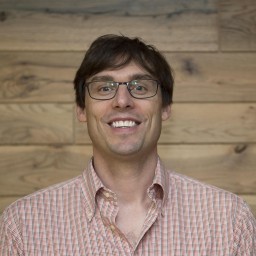
tim peterson
web programming-javascript, php, mysql, css, html-is my thang
Updated on September 15, 2022Comments
-
tim peterson 3 months
Has anyone figured out how to use twitter bootstrap collapse plugin where some text is shown (say, 100 characters) and you click a button to expand the text to show the rest of it?
Here's a JSFiddle that demonstrates the following issues I'm having:
- The hidden text is shown on a new line. Would be preferable if sentences wouldn't be visually disrupted.
- the
icon-plus-sign
indicator's display isn't toggled (doesn't go away on showing hidden text and re-appear vice versa).
Fully working HTML:
<link href="//netdna.bootstrapcdn.com/twitter-bootstrap/2.3.0/css/bootstrap-combined.min.css" rel="stylesheet"> <script src="//netdna.bootstrapcdn.com/twitter-bootstrap/2.3.0/js/bootstrap.min.js"></script> <div class="" data-toggle="collapse" data-target="#div1" > <span><b>Div1:</b> This is a sentence at the end of the first 100 characters that gets truncated </span> <i class='icon-plus-sign'></i> </div> <div id="div1" class="collapse" >such that characters 101 to infinity are not shown inline with the beginning of the sentence (<b>Div1</b>).</div> <div class="" data-toggle="collapse" data-target="#div2" > <span><b>Div2:</b>This is a sentence at the end of the first 100 characters that gets truncated </span> <i class='icon-plus-sign'></i> </div> <div id="div2" class="collapse" >such that characters 101 to infinity are not shown inline with the beginning of the sentence (<b>Div2</b>).</div>