TypeError: Failed to execute 'evaluate' on 'Document': The result is not a node set, and therefore cannot be converted using Xpath through Selenium
Solution 1
You are using the invalid xpath expression, use the below modified xpath :
IP_CLICK = browser.find_element_by_xpath("//span[text()='Gi2/0/20']");
IP_CLICK.click();
If there are multiple matches then use the indexing, I mean pass the matching index number in the below xpath :
xpath = "(//span[text()='Gi2/0/20'])[Matching index number goes here]";
IP_CLICK = browser.find_element_by_xpath(xpath);
IP_CLICK.click();
Solution 2
This error message...
TypeError: Failed to execute 'evaluate' on 'Document': The result is not a node set, and therefore cannot be converted to the desired type.
(Session info: chrome=72.0.3626.121) (Driver info: chromedriver=73.0.3683.20 (8e2b610813e167eee3619ac4ce6e42e3ec622017),platform=Windows NT 6.1.7601 SP1 x86_64)
...implies that the result of executing the xpath expression was not a node set.
Syntactically, the xpath expression you have used is a legit xpath expression which uses the text()
node as in parent_element/text()="Gi2/0/20"
following xpath v3.0.
Unfortunately Selenium implements xpath v1.0 which doesn't supports text()
nodes.
Additionally, click()
doesn't returns anything, so IP_CLICK
is not needed.
Solution
To click()
on the element, you can use either of the following solutions:
-
Xpath 1:
browser.find_element_by_xpath("//span[text()='Gi2/0/20']").click()
-
Xpath 2:
browser.find_element_by_xpath("//span[contains(., 'Gi2/0/20')]").click()
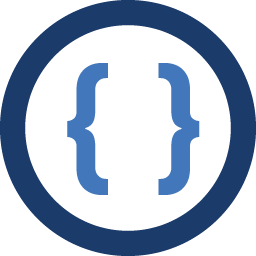
Admin
Updated on June 29, 2022Comments
-
Admin almost 2 years
Part of page source:
<span style="display:block; overflow:hidden; white-space: nowrap">Gi2/0/20</span>
Parts of the code:
from selenium import webdriver ... driver = webdriver.Chrome() ... IP_CLICK = browser.find_element_by_xpath('//span[@style="display:block; overflow:hidden; white-space: nowrap"]/text()="Gi2/0/20"').click()
I am trying to select an element in my web page with the
xpath
expression, but I'm getting the following error:InvalidSelectorException: invalid selector: Unable to locate an element with the xpath expression //span[@style="display:block; overflow:hidden; white-space: nowrap"]/text()="Gi2/0/20" because of the following error:
TypeError: Failed to execute 'evaluate' on 'Document': The result is not a node set, and therefore cannot be converted to the desired type. (Session info: chrome=72.0.3626.121) (Driver info: chromedriver=73.0.3683.20 (8e2b610813e167eee3619ac4ce6e42e3ec622017),platform=Windows NT 6.1.7601 SP1 x86_64)