TypeError: float() argument must be a string or a number, not 'NoneType'
24,234
This is the read()
function:
def read(self,cmd):
cmd_str = self.build_cmd_str(cmd,'')
try:
self.sp.write(cmd_str.encode())
self.sp.flush()
except Exception:
return None
return self.sp.readline().decode('UTF-8').replace("\r\n", "")
One of the things it can return is None
as you can see in the return None
line. If that happens, then your line:
float(self.read('T1'))
will fail, because it will try to convert None
into a float
, and that gives the error you're getting.
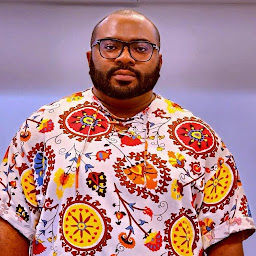
Author by
Tochukwu Anyaduba
Updated on June 14, 2020Comments
-
Tochukwu Anyaduba almost 4 years
i know that a lot of people have asked related questions but please help me out. I am trying to replicate an opensource temperature control lab i found online. I wanted to run it on Raspberry Pi. This is the error i keep getting:
Traceback (most recent call last): File "/home/pi/Desktop/Python/test_Temperature.py", line 14, in <module> print('Temperature 1: ' + str(a.T1) + ' degC') File "/home/pi/Desktop/Python/tclab.py", line 26, in T1 self._T1 = float(self.read('T1')) TypeError: float() argument must be a string or a number, not 'NoneType'
The code that generates it is this:
import tclab import numpy as np import time try: # Connect to Arduino a = tclab.TCLab() # Get Version print(a.version) # Temperatures print('Temperatures') print('Temperature 1: ' + str(a.T1) + ' degC') print('Temperature 2: ' + str(a.T2) + ' degC') # Turn LED on print('LED On') a.LED(100) # Turn on Heaters (0-100%) print('Turn On Heaters (Q1=90%, Q2=80%)') a.Q1(90.0) a.Q2(80.0) # Sleep (sec) time.sleep(60.0) # Turn Off Heaters print('Turn Off Heaters') a.Q1(0.0) a.Q2(0.0) # Temperatures print('Temperatures') print('Temperature 1: ' + str(a.T1) + ' degC') print('Temperature 2: ' + str(a.T2) + ' degC') # Allow user to end loop with Ctrl-C except KeyboardInterrupt: # Disconnect from Arduino a.Q1(0) a.Q2(0) print('Shutting down') a.close() # Make sure serial connection still closes when there's an error except: # Disconnect from Arduino a.Q1(0) a.Q2(0) print('Error: Shutting down') a.close() raise
I believe the code seeks to communicate with another python file with the below code:
import sys import time import numpy as np try: import serial except: import pip pip.main(['install','pyserial']) import serial from serial.tools import list_ports class TCLab(object): def __init__(self, port=None, baud=9600): if (sys.platform == 'darwin') and not port: port = '/dev/ttyACM1' def stop(self): return self.read('X') def version(self): return self.read('VER') @property def T1(self): self._T1 = float(self.read('T1')) return self._T1 @property def T2(self): self._T2 = float(self.read('T2')) return self._T2 def LED(self,pwm): pwm = max(0.0,min(100.0,pwm))/2.0 self.write('LED',pwm) return pwm def Q1(self,pwm): pwm = max(0.0,min(100.0,pwm)) self.write('Q1',pwm) return pwm def Q2(self,pwm): pwm = max(0.0,min(100.0,pwm)) self.write('Q2',pwm) return pwm # save txt file with data and set point # t = time # u1,u2 = heaters # y1,y2 = tempeatures # sp1,sp2 = setpoints def save_txt(self,t,u1,u2,y1,y2,sp1,sp2): data = np.vstack((t,u1,u2,y1,y2,sp1,sp2)) # vertical stack data = data.T # transpose data top = 'Time (sec), Heater 1 (%), Heater 2 (%), ' \ + 'Temperature 1 (degC), Temperature 2 (degC), ' \ + 'Set Point 1 (degC), Set Point 2 (degC)' np.savetxt('data.txt',data,delimiter=',',header=top,comments='') def read(self,cmd): cmd_str = self.build_cmd_str(cmd,'') try: self.sp.write(cmd_str.encode()) self.sp.flush() except Exception: return None return self.sp.readline().decode('UTF-8').replace("\r\n", "") def write(self,cmd,pwm): cmd_str = self.build_cmd_str(cmd,(pwm,)) try: self.sp.write(cmd_str.encode()) self.sp.flush() except: return None return self.sp.readline().decode('UTF-8').replace("\r\n", "") def build_cmd_str(self,cmd, args=None): """ Build a command string that can be sent to the arduino. Input: cmd (str): the command to send to the arduino, must not contain a % character args (iterable): the arguments to send to the command """ if args: args = ' '.join(map(str, args)) else: args = '' return "{cmd} {args}\n".format(cmd=cmd, args=args) def close(self): try: self.sp.close() print('Arduino disconnected successfully') except: print('Problems disconnecting from Arduino.') print('Please unplug and reconnect Arduino.') return True
I do not know my around python codes yet so a very clear 'for dummy class' explanation of the solution would really help. Thanks guys.
-
Acccumulation almost 6 yearsDo you understand the difference between a class and an object?
-
Tochukwu Anyaduba almost 6 yearsmaybe not in detail as a Pro should but i fairly understand the concept
-
-
Tochukwu Anyaduba almost 6 yearsThank you so much for your prompt response. Please, how do i correct it?
-
Tochukwu Anyaduba almost 6 yearsi changed None to 0. The program ran but only gave me the value 0. Please how do i effectively correct this?
-
nosklo almost 6 years@TochukwuAnyaduba that
try/except
construct is to catch errors in theself.sp.write
line. If some error occurs, the error is silenced and you getNone
(now0
with your edits) instead. In other words, you have some kind of error writing to the serial port, and the error is being silenced by the code, returning zero instead. Your device probably is not connected to the serial port or you have some other problem. I don't know if the code is complete but I can't find whereself.sp
is defined at all so maybe you're missing the definition? -
Tochukwu Anyaduba almost 6 yearsThank you once again. you are very correct. this is not the complete code. The files can be found here: github.com/jckantor/TCLab/tree/master/tclab
-
Tochukwu Anyaduba almost 6 yearsI ran the codes on Windows, it worked fine, but just not working on Raspberry pi
-
Tochukwu Anyaduba almost 6 yearsI finally got the code to work. Apparently, I was trying to communicate via serial while Arduino Leonardo was connected to my Pi via USB, I also was not using Arduino IDE meant for Linux so it kept crashing. Now everything runs smoothly. There is no problem with the code