TypeScript- How to add a Key Value pair to each object in an Array?
15,173
Solution 1
What's wrong with your code is that idx
will be the object not the index as you are using for...of
. Use a simple regular for
like:
for(let idx = 0; idx < data.length; idx++) {
data[idx].date = dates[idx];
}
Or use forEach
to loop one of the arrays and use the index it provides to get the value from the other array:
data.forEach((obj, i) => obj.date = dates[i]);
Solution 2
const result = data.map(({ name, age }, index) => ({ name, age, date: dates[index] }));
just map the array to the result.
Solution 3
Something like that:
let dates = ["10-12-18", "10-13-18", "10-14-18"];
let objs = [{"name":"One",
"age": "4"},
{"name":"Two",
"age": "5"},
{"name":"Three",
"age": "9"}
]
const result = objs.map((item, index) => {
item.Date = dates[index];
return item;
});
console.log(result);
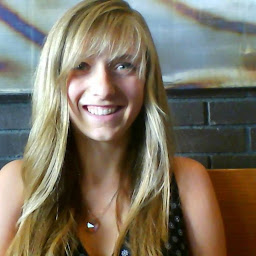
Author by
LaurenAH
Updated on June 27, 2022Comments
-
LaurenAH almost 2 years
I have an array of dates and an array of objects. I'd like to add the dates to the array of objects as a key value pair
{"Date": "10-12-18"}
.dates
:["10-12-18", "10-13-18", 10-14-18"]
data
:[ {"name":"One", "age": "4"}, {"name":"Two", "age": "5"}, {"name":"Three", "age": "9"} ]
I want something like...
[ {"name":"One", "age": "4", "Date": "10-12-18"}, ....
How can I do this in TypeScript? I'm used to normal JavaSCript and can't get it right.
Something I have so far:
for (let idx of data){ data[idx].date = dates[idx] }
Thanks!!
-
jonatjano over 5 yearsdo it like in JS, typescript should compile vanilla JS without problem
-
smac89 over 5 yearsCan you post the code you were using? Doing this in Javascript should be no different from doing it in Typescript. So if it wasn't working in JS, you are probably doing something wrong and typescript won't fix that
-
T.J. Crowder over 5 years"I'm used to normal JavaSCript and can't get it right." What have your non-right solutions looked like?
-
LaurenAH over 5 yearsEdited with my attempt
-
-
LaurenAH over 5 yearsPerfect and concise, thank you. I need to get the hang of transitioning into TypeSCript more. it's good to see the two things side by side!
-
ibrahim mahrir over 5 years@LaurenAH Actually this is still valid javascript as well.
-
ibrahim mahrir over 5 yearsBTW, using
map
is totally unecessary here as you are altering the original objects so a simpleforEach
will do.console.log(objs);
to see what I'm talking about.