UIActionSheet Dismiss when click/ tap outside
16,552
Solution 1
Hi refer below link: How to make a uiactionsheet dismiss when you tap outside eg above it?
http://splinter.com.au/how-to-allow-closing-a-uiactionsheet-by-tappi
Solution 2
An even more simpler solution would be to just add a Cancel button. This will automatically enable tap-background-to-dismiss: (Swift)
alertController.addAction(UIAlertAction(title: "Cancel",
style: .cancel,
handler: nil))
Solution 3
Try this magic:
UITapGestureRecognizer *tap = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(tapOut:)];
tap.cancelsTouchesInView = NO;
[actionSheet.window addGestureRecognizer:tap];
[tap release];
And this method:
-(void)tapOut:(UIGestureRecognizer *)gestureRecognizer {
CGPoint p = [gestureRecognizer locationInView:self.actionSheet];
if (p.y < 0) {
[self actionPickerDone:nil];
}
}
Solution 4
In SwiftUI
, when using ActionSheet
adding an Alert.Button.Cancel
achieves the desired result:
@State var isShowingActionSheet: Bool = false
@State var isShowingCamera: Bool = false
.actionSheet(isPresented: $isShowingActionSheet) {
ActionSheet(
title: Text("Send an image"),
buttons: [
.default(Text("Camera")) {
isShowingCamera = true
},
// This enables the "tap anywhere outside of the action sheet to dismiss it" behavior
.cancel()
]
)
}
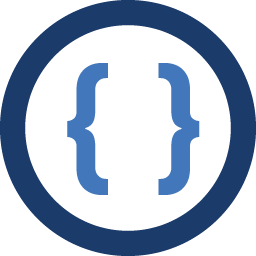
Author by
Admin
Updated on June 22, 2022Comments
-
Admin about 2 years
I'm novice in iPhone development. Can anyone tell me how to dismiss UIActionSheet control when i tapped outside of it?
In my actionSheet i have only datePicker control and it pops up over tab bar control now what i want whenever user click outside of it, it should dismiss instead of using actionSheet's cancel button. Regards
-
ram over 10 yearseven in iOS6, the delegate will not be called with a button index of -1 if clicked outside the action sheet. the documentation mentions that a button index of -1 will be passed if the cancel button index is not set.