UIkeyboard type
Solution 1
I'm affraid you cannot restrict the keyboard to only input alphabetic characters. The available keyboardTypes are listed in the UITextInputTraits protocol reference, and with even more detail in the header file:
typedef enum {
UIKeyboardTypeDefault, // Default type for the current input method.
UIKeyboardTypeASCIICapable, // Displays a keyboard which can enter ASCII characters, non-ASCII keyboards remain active
UIKeyboardTypeNumbersAndPunctuation, // Numbers and assorted punctuation.
UIKeyboardTypeURL, // A type optimized for URL entry (shows . / .com prominently).
UIKeyboardTypeNumberPad, // A number pad (0-9). Suitable for PIN entry.
UIKeyboardTypePhonePad, // A phone pad (1-9, *, 0, #, with letters under the numbers).
UIKeyboardTypeNamePhonePad, // A type optimized for entering a person's name or phone number.
UIKeyboardTypeEmailAddress, // A type optimized for multiple email address entry (shows space @ . prominently).
UIKeyboardTypeAlphabet = UIKeyboardTypeASCIICapable, // Deprecated
} UIKeyboardType;
I feel what you want is missing from the SDK. I would file a bug report with Apple requesting a new keyboard type, like the one you mentioned.
What is the solution for you besides filing a bug report and waiting for your new keyboard type to become available in the SDK? Checking the input in the textfield. This can be done by assigning the delegate property of the UITextField, and implementing the UITextFieldDelegate method textField:shouldChangeCharactersInRange:replacementString: and only return YES if the replacement string does not contain numbers.
HTH
Solution 2
the updated keyboard types
typedef NS_ENUM(NSInteger, UIKeyboardType) {
UIKeyboardTypeDefault, // Default type for the current input method.
UIKeyboardTypeASCIICapable, // Displays a keyboard which can enter ASCII characters, non-ASCII keyboards remain active
UIKeyboardTypeNumbersAndPunctuation, // Numbers and assorted punctuation.
UIKeyboardTypeURL, // A type optimized for URL entry (shows . / .com prominently).
UIKeyboardTypeNumberPad, // A number pad (0-9). Suitable for PIN entry.
UIKeyboardTypePhonePad, // A phone pad (1-9, *, 0, #, with letters under the numbers).
UIKeyboardTypeNamePhonePad, // A type optimized for entering a person's name or phone number.
UIKeyboardTypeEmailAddress, // A type optimized for multiple email address entry (shows space @ . prominently).
#if __IPHONE_4_1 <= __IPHONE_OS_VERSION_MAX_ALLOWED
UIKeyboardTypeDecimalPad, // A number pad with a decimal point.
#endif
#if __IPHONE_5_0 <= __IPHONE_OS_VERSION_MAX_ALLOWED
UIKeyboardTypeTwitter, // A type optimized for twitter text entry (easy access to @ #)
#endif
UIKeyboardTypeAlphabet = UIKeyboardTypeASCIICapable, // Deprecated
};
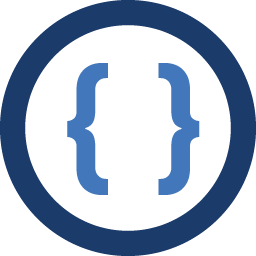
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to use only alphabetic keyboard, how to restrict it? I've used following statement but it doesn't hide the button that converts keyboard in numeric one
tempTextField.keyboardType = UIKeyboardTypeAlphabet;
-
teabot almost 15 yearsThere is an implementation of what @drvdijk suggests here: stackoverflow.com/questions/1013647/…