UILabel text not showing, but the "text" value is updating (shows in console)
Solution 1
Make sure you are setting the text
property on the main thread. If you don't, it will update the value, but won't redraw the label. All UIKit
elements must be updated only on the main thread.
Also you only need to create a second UILabel
if you aren't connecting to an IBOutlet
in Interface Builder.
Solution 2
If movieTitle
is connected to an Interface Builder UILabel;
then you should not be creating another instance:
self.movieTitle = [[UILabel alloc] init];
You are setting the text of the UILabel
not the one in the GUI.
Just delete that line, see below:
NSLog(@"movie details title in Movie object: %@", _movie.name);
[self.movieTitle setText:self.movie.name];
NSLog(@"movie details title in UILabel.text: %@",self.movieTitle.text);
Solution 3
This answer may not be your case, but you may find it helpful.
In my case, I put a label at the bottom of the login view controller to display the version of my app. When I run test the app, the label does not appear. The problem is because although the login .xib file is targeted for Retina 4 Full Screen, the Deployment Target (in App Summary) is 5.0, so when the app is run (on simulator), it actually the iPhone 5.0 simulator running, with a screen of 3.5". And since the label is at the bottom of the view, it's out of view region of 3.5" screen. To fix this, simply change the autosizing of the label.
Hope this helps.
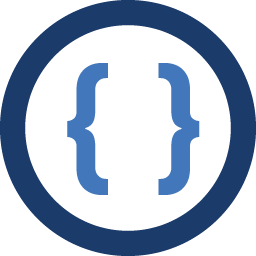
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have created a UILabel in my .storyboard file that has some initial text "Movie Title". I have connected that UILabel to a property defined in my ViewController files. For some reason the text will not display in the UI when I run the simulator. I've looked at similar questions, but I've not seen any response that helps.
I have the following property in my .h file
@property (strong, nonatomic) IBOutlet UILabel * movieTitle;
It's synthesized in my .m file
@synthesize movieTitle;
I then have some code that does the following:
NSLog(@"movie details title in Movie object: %@", _movie.name); self.movieTitle = [[UILabel alloc] init]; [self.movieTitle setText:self.movie.name]; NSLog(@"movie details title in UILabel.text: %@",self.movieTitle.text);
The console outputs the following
movie details title in Movie object: Transformers movie details title in UILabel.text: Transformers