UISwipeGestureRecognizer Swipe length
33,236
Solution 1
It's impossible to get a distance from a swipe gesture, because the SwipeGesture triggers the method where you could access the location exactly one time, when the gesture has ended.
Maybe you want to use a UIPanGestureRecognizer.
If it possible for you to use pan gesture you would save the starting point of the pan, and if the pan has ended calculate the distance.
- (void)panGesture:(UIPanGestureRecognizer *)sender {
if (sender.state == UIGestureRecognizerStateBegan) {
startLocation = [sender locationInView:self.view];
}
else if (sender.state == UIGestureRecognizerStateEnded) {
CGPoint stopLocation = [sender locationInView:self.view];
CGFloat dx = stopLocation.x - startLocation.x;
CGFloat dy = stopLocation.y - startLocation.y;
CGFloat distance = sqrt(dx*dx + dy*dy );
NSLog(@"Distance: %f", distance);
}
}
Solution 2
In Swift
override func viewDidLoad() {
super.viewDidLoad()
// add your pan recognizer to your desired view
let panRecognizer = UIPanGestureRecognizer(target: self, action: #selector(panedView))
self.view.addGestureRecognizer(panRecognizer)
}
@objc func panedView(sender:UIPanGestureRecognizer){
var startLocation = CGPoint()
//UIGestureRecognizerState has been renamed to UIGestureRecognizer.State in Swift 4
if (sender.state == UIGestureRecognizer.State.began) {
startLocation = sender.location(in: self.view)
}
else if (sender.state == UIGestureRecognizer.State.ended) {
let stopLocation = sender.location(in: self.view)
let dx = stopLocation.x - startLocation.x;
let dy = stopLocation.y - startLocation.y;
let distance = sqrt(dx*dx + dy*dy );
NSLog("Distance: %f", distance);
if distance > 400 {
//do what you want to do
}
}
}
Hope that helps all you Swift pioneers
Solution 3
func swipeAction(gesture: UIPanGestureRecognizer) {
let transition = sqrt(pow(gesture.translation(in: view).x, 2)
+ pow(gesture.translation(in: view).y, 2))
}
Solution 4
For those of us using Xamarin:
void panGesture(UIPanGestureRecognizer gestureRecognizer) {
if (gestureRecognizer.State == UIGestureRecognizerState.Began) {
startLocation = gestureRecognizer.TranslationInView (view)
} else if (gestureRecognizer.State == UIGestureRecognizerState.Ended) {
PointF stopLocation = gestureRecognizer.TranslationInView (view);
float dX = stopLocation.X - startLocation.X;
float dY = stopLocation.Y - startLocation.Y;
float distance = Math.Sqrt(dX * dX + dY * dY);
System.Console.WriteLine("Distance: {0}", distance);
}
}
Solution 5
You can only do it a standard way: remember the touch point of touchBegin and compare the point from touchEnd.
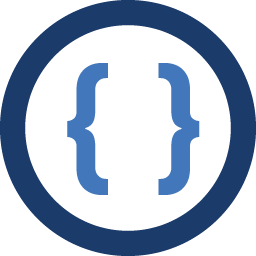
Author by
Admin
Updated on May 07, 2020Comments
-
Admin almost 4 years
Any idea if there is a way to get the length of a swipe gesture or the touches so that i can calculate the distance?
-
Jon over 10 yearsIf using a UIPanGestureRecognizer, the built in method - (CGPoint)translationInView:(UIView *)view works very well.
-
Fattie about 10 yearsYou'd certainly use translationInView here. Perhaps MB could edit the answer one day, since, it's such a high-marker! Eg stackoverflow.com/questions/15888276/… Rock on...
-
Apollo over 9 years@MatthiasBauch is this in inches?
-
GhostCat over 6 yearsPlease note: code only answers are discouraged. Please add a bit of explanation.
-
Radu Ursache about 6 yearsfor lazy people you should also declare
var startLocation = CGPoint()
in your class -
Hari Kunwar over 5 yearsIts the equation to calculate distance between two points. mathsisfun.com/algebra/distance-2-points.html
-
Mark Perkins over 4 yearsagreed @RaduUrsache, there is no way to store the start location in this solution above.