UML association and dependency
Solution 1
The short answer is: how any specific source language construct should be represented in UML is not strictly defined. This would be part of a standardized UML profile for the language in question, but these are sadly few and far between. Long answer follows.
In your example, I'm afraid I would have to say "neither", just to be difficult. A
has a member variable of type B
, so the relationship is actually an aggregation or a composition... Or a directed association. In UML, a directed association with a named target role is semantically equivalent to an attribute with the corresponding name.
As a rule of thumb, it's an aggregation if b
gets initialized in A
's constructor; it's a composition if it also gets destroyed in B
's destructor (shared lifecycle). If neither applies, it's an attribute / directed association.
If b
was not a member variable in A
, and the local variable b
was not operatoed on (no methods were called on it), then I would represent that as a dependency: A
needs B
, but it doesn't have an attribute of that type.
But f()
actually calls a method defined in B
. This to me makes the correct relationship a <<use>>
, which is a more specialized form of dependency.
Finally, an (undirected) association is the weakest form of link between two classes, and for that very reason I tend not to use them when describing source constructs. When I do, I usually use them when there are no direct source code relationships, but the two classes are still somehow related. An example of this might be a situation where the two are responsible for different parts of the same larger algorithm, but a third class uses them both.
Solution 2
It may be useful to see this question I asked: does an association imply a dependency in UML
My understanding is:
Association
public class SchoolClass{
/** This field, of type Bar, represents an association, a conceptual link
* between SchoolClass and Student. (Yes, this should probably be
* a List<Student>, but the array notation is clearer for the explanation)
*/
private Student[] students;
}
Dependency
public class SchoolClass{
private Timetable classTimetable;
public void generateTimetable(){
/*
* Here, SchoolClass depends on TimetableGenerator to function,
* but this doesn't represent a conceptual relationship. It's more of
* a logical implementation detail.
*/
TimetableGenerator timetableGen = new TimetableGenerator();
/*
* Timetable, however, is an association, as it is a conceptual
* relationship that describes some aspect of the data that the
* class holds (Remember OOP101? Objects consist of data and operations
* upon that data, associations are UMLs way or representing that data)
*/
classTimetable = timetableGen.generateTimetable();
}
}
Solution 3
Get it from Wiki: Dependency is a weaker form of relationship which indicates that one class depends on another because it uses it at some point of time. One class depends on another if the latter is a parameter variable or local variable of a method of the former. This is different from an association, where an attribute of the former is an instance of the latter.
So I think the case here is association, if B is a parameter variable or local variable of a method of the A, then they are dependency.
Solution 4
If you want to see the difference at the "code level", in an association between A and B, the implementation of A (or B or both depending on cardinalities, navigability,...) in an OO lang would include an attribute of type B.
Instead in a dependency, A would probably have a method where one of the parameters is of type B. So A and B are not linked but changing B would affect the dependant class A since maybe the way the A method manipulates the object B is no longer valid (e.g. B has changed the signature of a method and this induces a compile error in the class A)
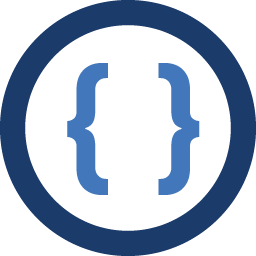
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
What is the difference between association and dependency? Can you give code examples? What is the relationship between class A and B?
class A { B *b; void f () { b = new B (); b->f(); delete b; } }