Unable to import opengl.gl in python on macos
Solution 1
This error is because Big Sur no longer has the OpenGL library nor other system libraries in standard locations in the file system and instead uses a cache. PyOpenGL uses ctypes to try to locate the OpenGL library and it fails to find it. Fixing ctypes in Python so that it will find the library is the subject of this pull request
https://github.com/python/cpython/pull/21241
So a future version of Python should resolve the problem.
To fix it now you can edit PyOpenGL file OpenGL/platform/ctypesloader.py changing line
fullName = util.find_library( name )
to
fullName = '/System/Library/Frameworks/OpenGL.framework/OpenGL'
Solution 2
Since I hate the idea of patching a Python package (plus it is really hard to manage that with use of Conda to install said package), I offer this kludge as a workaround for until Python gets its Big Sur (aka Big Nuisance) update:
try:
import OpenGL as ogl
try:
import OpenGL.GL # this fails in <=2020 versions of Python on OS X 11.x
except ImportError:
print('Drat, patching for Big Sur')
from ctypes import util
orig_util_find_library = util.find_library
def new_util_find_library( name ):
res = orig_util_find_library( name )
if res: return res
return '/System/Library/Frameworks/'+name+'.framework/'+name
util.find_library = new_util_find_library
except ImportError:
pass
Solution 3
It's better to change this to
fullName = "/System/Library/Frameworks/{}.framework/{}".format(name,name)
otherwise GLUT won't work. At least, this let me run the Cozmo SDK's 3d stuff again.
Solution 4
FYI, in my case it was:
fullName = '/Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/System/Library/Frameworks/OpenGL.framework/OpenGL'
Related videos on Youtube
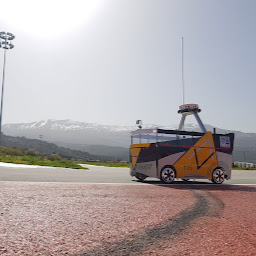
Iyad Boustany
Updated on June 05, 2022Comments
-
Iyad Boustany almost 2 years
I am using OpenGL to render a scene in python. My code works perfectly fine on windows but, for some reason, I'm having issues when importing opengl.gl on MacOS.
The issue arises when calling
from OpenGL.GL import ...
in both python scripts and the python console.More specifically here is the exact call in my script:
from OpenGL.GL import glGenBuffers, glBindBuffer, glBufferData, \ glGenVertexArrays, glBindVertexArray, glEnableVertexAttribArray, glVertexAttribPointer, \ glDrawArrays, glUseProgram, glEnable, glDisable, \ GL_ARRAY_BUFFER, GL_STATIC_DRAW, GL_DEPTH_TEST, \ GL_FLOAT, GL_FALSE, \ GL_TRIANGLES, GL_LINES, GL_LINE_STRIP
This results in the following error:
Traceback (most recent call last): File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/darwin.py", line 35, in GL return ctypesloader.loadLibrary( File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/ctypesloader.py", line 36, in loadLibrary return _loadLibraryWindows(dllType, name, mode) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/ctypesloader.py", line 89, in _loadLibraryWindows return dllType( name, mode ) File "/usr/local/Cellar/[email protected]/3.8.5/Frameworks/Python.framework/Versions/3.8/lib/python3.8/ctypes/__init__.py", line 373, in __init__ self._handle = _dlopen(self._name, mode) OSError: ('dlopen(OpenGL, 10): image not found', 'OpenGL', None) During handling of the above exception, another exception occurred: Traceback (most recent call last): File "/Users/iyadboustany/Desktop/lensmaster/Lensmaster.py", line 18, in <module> from MainWindow import MainWindow File "/Users/iyadboustany/Desktop/lensmaster/MainWindow.py", line 14, in <module> from Robot import Robot File "/Users/iyadboustany/Desktop/lensmaster/Robot.py", line 8, in <module> from Graphics.Scene import DHNode File "/Users/iyadboustany/Desktop/lensmaster/Graphics/Scene.py", line 13, in <module> from OpenGL.GL import glGenBuffers, glBindBuffer, glBufferData, \ File "/usr/local/lib/python3.8/site-packages/OpenGL/GL/__init__.py", line 3, in <module> from OpenGL import error as _error File "/usr/local/lib/python3.8/site-packages/OpenGL/error.py", line 12, in <module> from OpenGL import platform, _configflags File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/__init__.py", line 36, in <module> _load() File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/__init__.py", line 33, in _load plugin.install(globals()) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/baseplatform.py", line 97, in install namespace[ name ] = getattr(self,name,None) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/baseplatform.py", line 15, in __get__ value = self.fget( obj ) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/darwin.py", line 62, in GetCurrentContext return self.CGL.CGLGetCurrentContext File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/baseplatform.py", line 15, in __get__ value = self.fget( obj ) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/darwin.py", line 45, in CGL def CGL(self): return self.GL File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/baseplatform.py", line 15, in __get__ value = self.fget( obj ) File "/usr/local/lib/python3.8/site-packages/OpenGL/platform/darwin.py", line 41, in GL raise ImportError("Unable to load OpenGL library", *err.args) ImportError: ('Unable to load OpenGL library', 'dlopen(OpenGL, 10): image not found', 'OpenGL', None)
Notes:
- Running glxgears works just fine.
- I'm running macOS Big Sur beta (20A5343i)
- I'm using python 3.8.5
- I installed opengl using pip:
pip3 install PyOpenGL PyOpenGL_accelerate
-
Julian A. over 3 yearsWhere is PyOpenGL located?
-
yudhiesh over 3 yearsYes how would I access the file?
-
aeengineer over 3 yearsHow would I obtain the correct filepath for my specific case? Please advise
-
imflash217 about 3 years@JulianA. @yudhiesh @aeengineer you can find the file at this location
~/anaconda3/lib/python3.7/site-packages/OpenGL/platform/ctypesloader.py
or similar paths to your python installation -
YuvGM about 3 yearsIndeed fixed my problems.
-
fishbacp over 2 years@TomGoddard --This was so helpful. Thanks!
-
aryan singh about 2 yearshi i am having the same issue is there a fix for this on mac m1,
-
aryan singh about 2 yearscontent of (base) [~/Downloads/resources]$ ls /System/Library/Frameworks/OpenGL.framework/ Libraries Resources Versions , <- are these, and adding that file path did not help as there was no folder OpenGl in there
-
Konchog almost 2 yearsPython 3.10 fixes the whole mess.