Unable to Merge Dex - Android Studio 3.0
Solution 1
Add an explicit dependency to play-services-auth
along with your firebase-ui-auth
dependency:
// FirebaseUI for Firebase Auth
compile 'com.firebaseui:firebase-ui-auth:3.1.0'
compile 'com.google.android.gms:play-services-auth:11.4.2'
This is because firebase-ui-auth
has a transitive dependency to play-services-auth
and must be used with the corresponding version of play-services-auth
. Please see this explanation.
firebase-ui-auth
|--- com.google.firebase:firebase-auth
|--- com.google.android.gms:play-services-auth
Earlier versions of the Gradle build tool did not include transitive dependencies so now versions can conflict with other play-services
versions.
My Issue Explained and Answered (In case anyone wants to know)
When you upgrade to Android Studio 3.0 and update the gradle build tool version to 3.0.0, compiling of dependencies is now done differently than in earlier versions.
I recently encountered the same issue. I was using these dependencies which worked fine through Gradle version 2.3.3:
implementation 'org.apache.httpcomponents:httpmime:4.3.6'
implementation 'org.apache.httpcomponents:httpclient-android:4.3.5.1'
After the upgrade to gradle-build-version 3.0.0, I got the same error. Digint into it, I found that the transitive dependency of httpmime
conflicted with the file httpclient-android
was including.
Description
Let me explain this in detail. Earlier, while using gradle-tool-version 2.3.3, I was using httpclient-android
to fetch and use the class named org.apache.http.entity.ContentType.java
Expanding the transitive dependencies of org.apache.httpcomponents:httpmime:4.3.6
showed that it has org.apache.httpcomponents:httpcore:4.3.6
which is the same package I wanted to use. But while compiling or syncing the build, it was excluding org.apache.http.entity.ContentType.java
so I needed to add this dependency which includes ContentType.java
:
implementation 'org.apache.httpcomponents:httpclient-android:4.3.5.1'
Everything worked fine after that.
Once I upgraded the gradle-build-version to 3.0.0, things changed. It now included all transitive dependencies. So while compiling with the latest Android Studio with gradle-build-tool version 3.0.0, my ContentType.java
was being compiled twice. Once from org.apache.httpcomponents:httpcore:4.3.6
(which is an implicit transitive dependency of httpmime
) and again from org.apache.httpcomponents:httpclient-android:4.3.5.1
which I was using earlier.
To resolve this issue I had to remove the existing org.apache.httpcomponents:httpclient-android:4.3.5.1
dependency as httpmime
would itself fetch the relevant class required for my application.
The solution for my situation: only use required dependencies and remove the httpclient-android
implementation 'org.apache.httpcomponents:httpmime:4.3.6'
Note that this is just the case for me. You'll need to dig into your own dependencies and apply the solution accordingly.
Solution 2
First of all I enabled multidex as suggested in previous comments.
Then, if the error continues, open the Gradle Console (click on "Show Console Output" icon at left of "Messages" section) and click on the link to recompile with Debug/Info/Stack options. This will show further details about the error.
In my case, the error "Unable to merge dex" was caused by duplicate entries in "com.jakewharton.picasso:picasso2-okhttp3-downloader:1.1.0".
I manually removed the conflicting library from my project and executed the "Rebuild Project" (forcing to reload the library). This solved the issue.
Solution 3
Check out dependencies in your build.gradle (app) if you're using 2 (or more) libraries with the same name and different version. For example (in my case):
implementation files('src/main/libs/support-v4-24.1.1.jar')
implementation 'com.android.support:support-v4:27.0.2'
Remove one, then clean and rebuild. Also note that dependencies is outside buildscript.
Solution 4
I had this error:
com.android.builder.dexing.DexArchiveMergerException: Unable to merge dex
and ended up changing back my gradle in order to fix this issue.
app\build.gradle
android {
compileSdkVersion 25
//buildToolsVersion '26.0.2'
buildToolsVersion '25.0.3'//<< Changed back to old version before my studio 3.0 update
defaultConfig { ....
.\build.gradle
buildscript {
repositories {
jcenter()
google()
}
dependencies {
classpath 'com.android.tools.build:gradle:2.3.3' //<< Changed back to old version before my studio 3.0 update
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
It's not ideal as it's back dating, but it's something which worked for me and should get me there until a possible patch is released.
Solution 5
android {
defaultConfig {
multiDexEnabled true
}
}
add this line to the :gradle
file
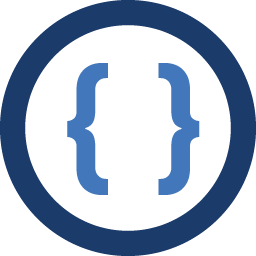
Admin
Updated on March 06, 2021Comments
-
Admin over 3 years
When I updated my Android Studio to 3.0 in the stable channel and ran the project, I started getting the below error.
Error:Execution failed for task ':app:transformDexArchiveWithExternalLibsDexMergerForDebug'. com.android.builder.dexing.DexArchiveMergerException: Unable to merge dex
I tried cleaning and rebuilding the project, but it didn't work. Any help will be appreciated.
Project level build.gradle
buildscript { repositories { jcenter() google() } dependencies { classpath 'com.android.tools.build:gradle:3.0.0' classpath 'com.google.gms:google-services:3.1.0' // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { jcenter() google() } } task clean(type: Delete) { delete rootProject.buildDir }
App level build.gradle
apply plugin: 'com.android.application' android { compileSdkVersion 26 buildToolsVersion '26.0.2' defaultConfig { applicationId "com.med.app" minSdkVersion 21 targetSdkVersion 26 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" resConfigs "auto" multiDexEnabled true } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', { exclude group: 'com.android.support', module: 'support-annotations' }) compile 'com.android.support.constraint:constraint-layout:1.0.2' testCompile 'junit:junit:4.12' //appcompat libraries compile 'com.android.support:appcompat-v7:26.1.0' compile 'com.android.support:design:26.1.0' //butterknife compile 'com.jakewharton:butterknife:8.8.1' annotationProcessor 'com.jakewharton:butterknife-compiler:8.8.1' //picasso compile 'com.squareup.picasso:picasso:2.5.2' //material edittext compile 'com.rengwuxian.materialedittext:library:2.1.4' // Retrofit & OkHttp & and OkHttpInterceptor & gson compile 'com.squareup.retrofit2:retrofit:2.3.0' compile 'com.squareup.retrofit2:converter-gson:2.3.0' compile 'com.google.code.gson:gson:2.8.2' compile 'com.squareup.okhttp3:logging-interceptor:3.8.0' // FirebaseUI for Firebase Auth compile 'com.firebaseui:firebase-ui-auth:3.1.0' } apply plugin: 'com.google.gms.google-services'
I have tried all the answers given but I am unable to solve this error. Please help.
-
Russ Wheeler over 6 yearsWhere exactly did you see duplicate entries? In a build.gradle file? The actual jar in a folder? Thanks
-
AutonomousApps over 6 yearsI'm getting
com.android.dex.DexIndexOverflowException
, despite the fact I have multidex enabled and my project built fine before updating to Android Gradle Plugin 3.0. -
Admin over 6 yearsI did as mentioned and got the following: Caused by: com.android.dex.DexException: Multiple dex files define Lcom/google/firebase/iid/zzd; I tried removing FirebaseUI library, rebuilding the project and then again copying the dependency. But it's not working.
-
Harish Rana over 6 yearsExactly, I was also stuck with similar issue. One of my project dependency and its transitive dependent library had a dependency on 'com.android.volley'. So this new gradle was having having issues while merging dex files. I fixed it by adding "exclude group: 'com.android.volley' in my gradle file.
-
dan_flo10 over 6 yearsEres una pistola
-
Pardeep Sharma over 6 years@Bhavesh Patadiya, Please help me here (stackoverflow.com/questions/46976279/…). I just got stuck and unable to resolve it.
-
Erhannis over 6 yearsIt took a long time to pinpoint and eliminate the duplicate dependencies (which I feel gradle ought to do automatically), but recompiling with stack info (showing me which class was duplicated) was the tool I needed to be able to fix it at all.
-
pirho over 6 yearsWhile this may answer the question, it is better to explain the essential parts of the answer and possibly what was the problem with OPs code.
-
Alexander over 6 yearsWhile this code may answer the question, providing additional context regarding how and why it solves the problem would improve the answer's long-term value.
-
xarlymg89 over 6 yearsLucky me that your explanation happen to be related with org.apache.httpcomponents:httpclient-android and org.apache.httpcomponents:httpmime . I was getting crazy to find out about it. Thanks!
-
Denys Kniazhev-Support Ukraine about 6 years
you need to dig into the classes your are having an issue with
But how did you find out which classes you've been having an issue with? Did you run./gradlew dependencies
and go through all transitive dependencies (marked asxxx -> yyy
in the output)? -
Midou Dz about 6 yearsthis is dependencies
-
Midou Dz about 6 yearsdependencies { compile 'com.google.android.gms:play-services:12.0.0' compile files('libs/dagger-1.2.2.jar') compile files('libs/javax.inject-1.jar') compile files('libs/nineoldandroids-2.4.0.jar') compile files('libs/support-v4-19.0.1.jar') }
-
Cyrus about 6 yearsI think you'd better edit your answer again for others understand your idea clearly